As mentioned by others:
Graphics2D
extends Graphics
and inherits all the methods of the parent class.
- The
Graphics
object passed to Swing components should be a Graphics2D
instance.
In this code, I side step the 2nd assumption by painting to a BufferedImage
displayed in a JLabel
. In the top of the GUI we can see the 'complete' image obtained from Example images for code and mark-up Q&As. In the bottom the code crops the image to just the 'black' king, rotates it and paints a clipped version (repeatedly).
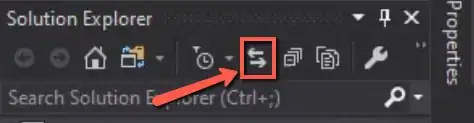
import java.awt.*;
import java.awt.event.*;
import java.awt.image.BufferedImage;
import javax.swing.*;
import java.net.URL;
import java.util.Random;
import javax.imageio.ImageIO;
class ClippedRotatedImage {
public static void main(String[] args) throws Exception {
URL url = new URL("https://i.stack.imgur.com/memI0.png");
final BufferedImage bi = ImageIO.read(url);
Runnable r = new Runnable() {
@Override
public void run() {
JPanel gui = new JPanel(new BorderLayout());
gui.add(new JLabel(
new ImageIcon(bi)),
BorderLayout.PAGE_START);
final BufferedImage bi2 = new BufferedImage(
400,
150,
BufferedImage.TYPE_INT_RGB);
final JLabel l = new JLabel(
new ImageIcon(bi2), SwingConstants.CENTER);
gui.add(l, BorderLayout.CENTER);
ActionListener animatonListener = new ActionListener() {
Random rnd = new Random();
@Override
public void actionPerformed(ActionEvent e) {
Graphics2D g = bi2.createGraphics();
int x = rnd.nextInt(bi2.getWidth());
int y = rnd.nextInt(bi2.getHeight());
double theta = rnd.nextDouble()*2*Math.PI;
g.rotate(theta);
g.setClip(x,y,64,64);
g.drawImage(bi,x,y,null);
g.dispose();
l.repaint();
}
};
Timer timer = new Timer(50, animatonListener);
timer.start();
JOptionPane.showMessageDialog(null, gui);
timer.stop();
}
};
// Swing GUIs should be created and updated on the EDT
// http://docs.oracle.com/javase/tutorial/uiswing/concurrency
SwingUtilities.invokeLater(r);
}
}