I'd recommend using UIActivityViewController as this should show all of the apps on the device which are capable of sending the link. This will only work for setting the contents of the message though; unfortunately, it doesn't seem like it's possible to set the recipient using this technique.
NSString *text = @"Click on this link!!";
NSURL *url = [NSURL URLWithString:@"http://google.com"];
NSArray *itemsToShare = @[text, url];
UIActivity *activity = [[UIActivity alloc] init];
NSArray *applicationActivities = [[NSArray alloc] initWithObjects:activity, nil];
UIActivityViewController *activityVC =
[[UIActivityViewController alloc] initWithActivityItems:itemsToShare
applicationActivities:applicationActivities];
[self presentViewController:activityVC
animated:YES
completion:nil];
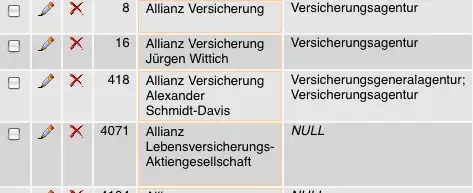
If you know you definitely want to send an SMS (rather than letting the user decide) then there are a few other options.
MFMessageComposeViewController
The following web page does a really good job of explaining how to do this: http://www.appcoda.com/ios-programming-send-sms-text-message
Open SMS: URL
It's possible to show the SMS composer using a simple "SMS:" link but you can only pre-populate the recipient's phone number, not the message, so this probably won't be good enough for what you need. For example:
[[UIApplication sharedApplication] openURL:[NSURL URLWithString:@"sms:1-408-555-1212"]];
https://developer.apple.com/library/ios/featuredarticles/iPhoneURLScheme_Reference/SMSLinks/SMSLinks.html