EDIT - I updated some of the references in the saveImgData:fromURL to match the parameters passed in the method. I used a variation of this method within an NSURL protocol to intercept requests and cache specific ones. Some of the entity parameters may not apply to your question. Just disregard those (such as encoding/lastModified/mimeType/response).
To save an image to CoreData give this a try.
First, download your image. A user can enter the url in a text entry field. (The example listed by andrewbuilder will work just fine)
NSURL *imgURL = [NSURL URLWithString:myImgURL];
NSData *imgData = [NSData dataWithContentsOfURL:imgURL];
//a better way would be to do this asynchronously. Google "Lazy Image Loading" and
//you should find a suitable example app from Apple
Setup your CoreData entity to store the image data. Here is a sample that stores more than just the image binary data.
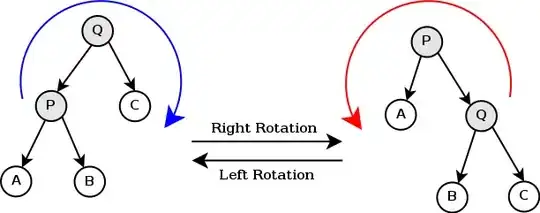
Then save your image data directly to CoreData.
I have a CoreData manager class but you may have your CoreData stack in AppDelegate which is how Apple sets them up.
-(void)saveImgData:(NSData*)myImgData fromURL:(NSString*)urlStr{
//If you have a CoreData manager class do something like this
CoreDataManager *cdm = [CoreDataManager defaultManager];
//Use private queue concurrency type for background saving
NSManagedObjectContext *ctx = [[NSManagedObjectContext alloc]initWithConcurrencyType:NSPrivateQueueConcurrencyType];
//Set up the parent context, in this case is the mainMOC
//If you are using basic CoreData it would be the managedObjectContext on your AppDelegate
ctx.parentContext = cdm.mainMOC;
[ctx performBlock:^{
//performBlock executes on a background thread
CachedURLResponse *cacheResponse = [NSEntityDescription insertNewObjectForEntityForName:@"CachedURLResponse" inManagedObjectContext:ctx];
cacheResponse.relativeURLHash = [NSNumber numberWithInteger:[urlStr hash]];
cacheResponse.data = [myImgData copy];
cacheResponse.response = [NSKeyedArchiver archivedDataWithRootObject:self.response];
cacheResponse.url = [urlStr copy];
cacheResponse.timestamp = [NSDate date];
cacheResponse.mimeType = [self.response.MIMEType copy];
cacheResponse.encoding = [self.response.textEncodingName copy];
if ([self.headers objectForKey:@"Last-Modified"])
cacheResponse.lastModified = [self.headers objectForKey:@"Last-Modified"];
NSError *__block error;
if (![ctx save:&error])
NSLog(@"Error, Cache not saved: %@", error.userInfo);
[cdm.mainMOC performBlockAndWait: ^{
[cdm saveContext];
}];
}];
}
My CoreDataManager.h looks like this:
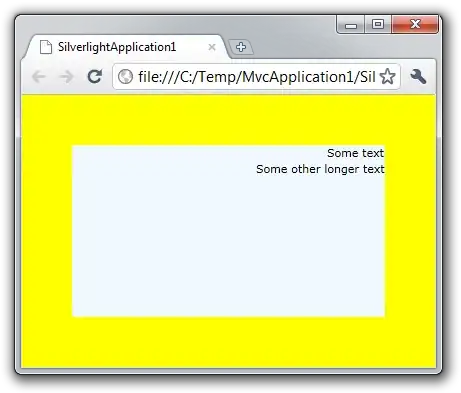
and my CoreDataManager.m file looks like this:
#import "CoreDataManager.h"
#import <CoreData/CoreData.h>
@implementation CoreDataManager
@synthesize mainMOC = _mainMOC,
managedObjectModel = _mom,
persistentStoreCoordinator = _psc
;
+(CoreDataManager*)defaultManager
{
static CoreDataManager *_defaultMgr = nil;
static dispatch_once_t oncePred;
dispatch_once(&oncePred, ^{
_defaultMgr = [[CoreDataManager alloc] init];
});
return _defaultMgr;
}
-(id)init
{
if (self = [super init])
{
NSError *error = nil;
NSURL *storeURL = [[self applicationDocumentsDirectory] URLByAppendingPathComponent:@"MyDatabase.sqlite"];
NSURL *modelURL = [[NSBundle mainBundle] URLForResource:@"MyDataModelName" withExtension:@"momd"];
_mom = [[NSManagedObjectModel alloc] initWithContentsOfURL:modelURL];
_psc = [[NSPersistentStoreCoordinator alloc] initWithManagedObjectModel:[self managedObjectModel]];
if (![_psc addPersistentStoreWithType:NSSQLiteStoreType configuration:nil URL:storeURL options:nil error:&error])
NSLog(@"Unresolved error %@, %@", error, [error userInfo]);
_mainMOC = [[NSManagedObjectContext alloc] initWithConcurrencyType:NSMainQueueConcurrencyType];
[_mainMOC setPersistentStoreCoordinator:self.persistentStoreCoordinator];
}
return self;
}
- (void)saveContext
{
NSError *error = nil;
if (self.mainMOC != nil && ([self.mainMOC hasChanges] && ![self.mainMOC save:&error])) {
NSLog(@"CoreData save error: %@, %@", error, [error userInfo]);
}
}
#pragma mark - Core Data Stack
- (NSManagedObjectContext *)mainMOC
{
return _mainMOC;
}
- (NSManagedObjectModel *)managedObjectModel
{
return _mom;
}
- (NSPersistentStoreCoordinator *)persistentStoreCoordinator
{
return _psc;
}
- (NSURL *)applicationDocumentsDirectory
{
return [[[NSFileManager defaultManager] URLsForDirectory:NSDocumentDirectory inDomains:NSUserDomainMask] lastObject];
}
@end
Hope that gives you a good starting point. Google "CoreData Class Reference" for some really good reading on the topic.