This is how you populate a ListBox
with items.
For i = 1 to 5
With me.ListBox1
.AddItem i
End With
Next
So assuming you have ListBox1
in UserForm1
, this will result to:
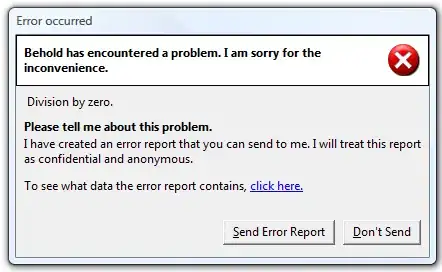
Let's say you want to replace 5
by 6
, you use .List
property like this:
Me.ListBox1.List(4, 0) = 6
Syntax: expression.List(pvargIndex, pvargColumn)
Again, when you use .List
property, Index
and Column
is always 1 less the actual Item
and Column
.
Since we want to replace 5
by 6
in a ListBox
with 1 Column and 5 items in it, the Index
of 5
is 4 and it's Column
is 0.
The result would be:
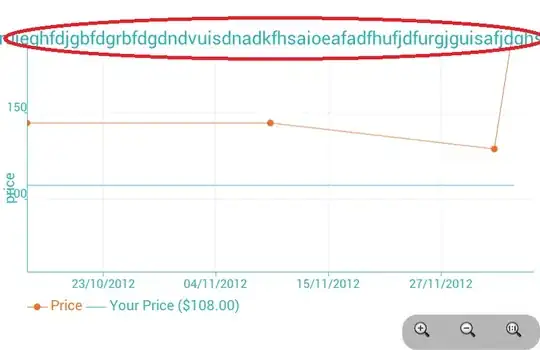
Now, what if you have multiple-column ListBox
and you want to populate those Columns
.
Say Column
2 of our ListBox1
, we use this code.
For i = 1 To 5
With Me.ListBox1
.List(i - 1, 1) = "Item" & i
End With
Next
Take note of the Index
which is i - 1
and the Column
which is 1
(actual column count -1)
The result would be:
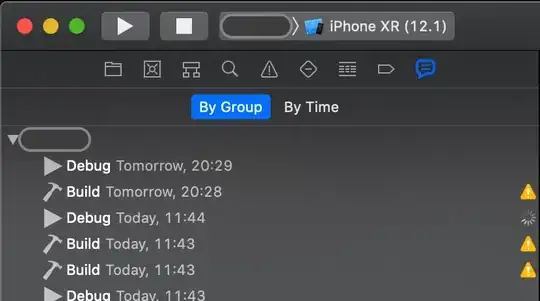
So let's go back to your question.
This line:
If ws.cells(A,B).value = "1" then .ListBox1.List(.ListBox1.ListIndex,5) = Checkbox.name
will fail since you are trying to populate Column 6
of your ListBox1
which I believe doesn't exist.
Also you used .ListIndex
property which returns the currently selected item index.
I don't know where you are executing your code but initially, .ListIndex
value is -1
since it will only have value on runtime when the user get to select an Item.
Now these lines:
.list(.listcount -1, 1) = ws.cells(C,D).value
.list(.listcount -1, 1) = ws.cells(E,F).value
actually just replaces the last item second column value.
First it passes ws.cells(C,D).Value
to it then overwrites it with ws.cells(E,F).value
.
Hope this anwers some of your questions a bit.