This may be well answered already, but I would like to share my resolution for those who still looking for a different approach.
If you would like to convert string dot notation to a string JSON, here's my approach.
function dotToJson({ notation, inclusive = true, value = null }) {
const fragments = notation.split(".").reverse();
if (!inclusive) {
fragments.pop();
}
console.log(fragments);
return fragments.reduce((json, fragment) => {
if (isNaN(fragment)) {
return `{ "${fragment}": ${json} }`
}
let fill = "";
if (Number(fragment) > 0) {
for (let i = 0; i < fragment; i++) {
fill += "null, "
}
}
return `[${fill}${json}]`;
}, JSON.stringify(value));
};
Attribute |
Meaning |
notation |
Dot notation string |
inclusive |
Include the root fragment |
value |
Default value for leaf |
You can see the results here, I tested it using Quokka.js
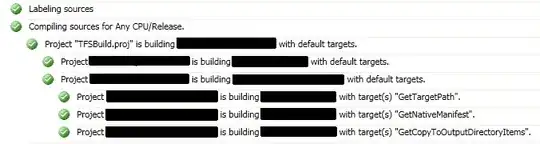
NOTE: Additionally to this, thiss may help to update objects because you can use spread operator with the parsed version of the JSON