Your original regex:
(?P<item>.+?)\-(?P<food>.*)\[.*?(?P<extra>\d+(\.\d+)?).*\].*\[.*?(?P<required>\d+(\.\d+)?).*\]

Debuggex Demo
Your problems are mostly due to the fact that you are searching for any character, instead of specific ones (digits and static strings). For example: Why do you use
(?P<item>.+?)
if it's only going to be numbers? Change it to
(?P<item>[0-9]+?)
and the '+?':reluctant operator is not necessary in this case, since you always want the entire number. That is, the next portion of the match will not be in the middle of that number.
In addition, this should be anchored to line (input) start:
^(?P<item>[0-9]+?)
You don't need to escape the dash (although it doesn't hurt).
^(?P<item>[0-9]+?)-
Your food group (heh) is the most complicated part
(?P<food>.*)
It doesn't just contain any character. Based on your demo input, it only has letters, spaces, numbers, and parens. So search just for them:
(?P<food>[\w0-9 ()]+)
Here's what we have so far:
^(?P<item>[0-9]+?)- (?P<food>[\w0-9 ()]+)
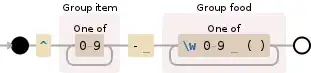
Debuggex Demo
You'll see that this also matches the cost part (which is completely missing from your regex...I assume that's just an oversight).
So add the cost, which is
(
- a number
[space]DOLLARS)
But only capture the number:
^(?P<item>[0-9]+?)- (?P<food>[\w0-9 ()]+) \((?P<cost>[0-9]+) DOLLARS\)
The rest of your regex works fine, actually, and it can be added to the end as is:
\[.*?(?P<extra>\d+(\.\d+)?).*\].*\[.*?(?P<required>\d+(\.\d+)?).*\]
I'd recommend, however, changing the .*?
to EXTRA[space]
if indeed that text is always found there (and again, no need for reluctance in this case). Same with [space]COUNT
, ;
and REQUIRED[space]
. The more you narrow things down, the easier your regex will be to debug--assuming your input is indeed that restricted.
Here's the final version (with an end-of-line anchor as well):
^(?P<item>[0-9]+?)- (?P<food>[\w0-9 ()]+) \((?P<cost>[0-9]+) DOLLARS\) \[EXTRA (?P<extra>\d+(\.\d+)?) COUNT\]; \[REQUIRED (?P<required>\d+(\.\d+)?) COUNT\]$

Debuggex Demo
Before analyzing your regex, this is what I came up with:
(?P<item>[0-9]+)- (?P<food>[\w ()]+) \((?P<cost>[0-9]+) DOLLARS\) \[EXTRA (?P<extra>[0-9]+) COUNT\]; \[REQUIRED (?P<required>[0-9]+) COUNT\]

Debuggex Demo
All these links came from the Stack Overflow Regular Expressions FAQ.