By 2019, the accepted answer is erroneous and you can make a radial gradient by setting its type property to .radial.
On thing important is that the startPoint is the coordinate of the center of the ellipse or circle and the endPoint are the dimensions of the outer eclipse or circle. All coordinates have to be expressed in unit-based (aka 1.0 is 100%).
The following Swift example will draw an ellipse positioned at the center of the frame and the radiuses set to match the frame:
let g = CAGradientLayer()
// We want a radial gradient
g.type = .radial
g.colors = [UIColor.clear.cgColor, UIColor.black.cgColor]
let center = CGPoint(x: 0.5, y: 0.5)
g.startPoint = center
let radius = 1.5
g.endPoint = CGPoint(x: radius, y: radius)
The above example can be used to add a vignette to a view for example (see the image).
Note that the radius can be larger than the frame, and the center can be placed outside the frame as well by specifying coordinates greater than 1 or lower than -1.
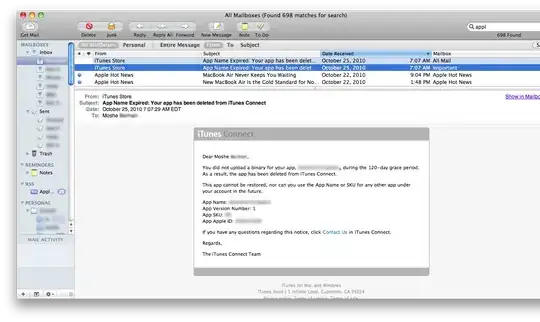