I would also be interested in knowing this. So far, poking around the cert, I did the following:
id data = [keychainDictionary objectForKey:@"issr"];
You can then set a breakpoint at this line, and as you step over it, select the "data" variable in the debug window left panel. Select "watch memory of *data", and you will see a bunch of garbage with real strings from that data. I don't know how to proceed from there.
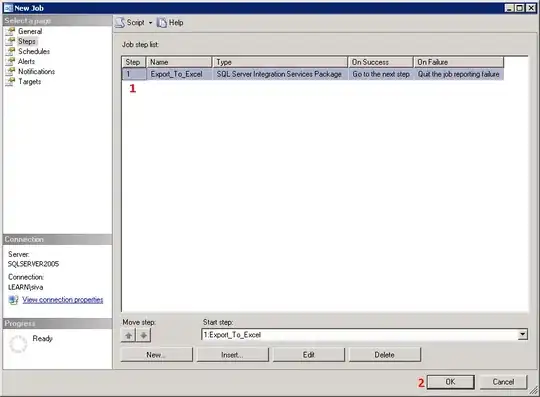
Complete method that gets all keychain items and loads them in the table view:
-(void)loadDataSource
{
//enumerate all items in keychain http://stackoverflow.com/questions/10966969/enumerate-all-keychain-items-in-my-ios-application
NSMutableDictionary *query = [NSMutableDictionary dictionaryWithObjectsAndKeys:
(__bridge id)kCFBooleanTrue, (__bridge id)kSecReturnAttributes,
(__bridge id)kSecMatchLimitAll, (__bridge id)kSecMatchLimit,
nil];
NSArray *secItemClasses = [NSArray arrayWithObjects:
(__bridge id)kSecClassGenericPassword,
(__bridge id)kSecClassInternetPassword,
(__bridge id)kSecClassCertificate,
(__bridge id)kSecClassKey,
(__bridge id)kSecClassIdentity,
nil];
NSMutableArray* results = [NSMutableArray array];
for(int i = 0; i < (int)secItemClasses.count;i++)
{
[results addObject:[NSMutableArray array]];
}
for (id secItemClass in secItemClasses) {
[query setObject:secItemClass forKey:(__bridge id)kSecClass];
CFTypeRef result = NULL;
SecItemCopyMatching((__bridge CFDictionaryRef)query, &result);
// NSLog(@"%@", (__bridge id)result);
if (result != NULL)
{
NSMutableArray* thisSection = results[[secItemClasses indexOfObject:secItemClass]];
[thisSection addObject:(__bridge id)result];
// [results addObject:(__bridge id)result];
CFRelease(result);
}
for(NSArray* object in results[[secItemClasses indexOfObject:secItemClass]])
{
DLog(@"object is of class: %@",[[object class] description]);
for (NSDictionary* innerObject in object)
{
DLog(@"object is of class: %@",[[innerObject class] description]);
}
}
}
self.datasource = results;
[self.tableView reloadData];
}
//this is the description, you can assign it to a text label in a table view cell
-(NSMutableString*)descriptionForObject:(NSDictionary*)object
{
NSMutableString* string = [[NSMutableString alloc] initWithCapacity:1024];
// https://developer.apple.com/library/mac/documentation/security/Reference/keychainservices/Reference/reference.html
//search for kSecAlias for a list of codes
if(object[@"labl"] != nil)
{
[string appendString:[NSString stringWithFormat:@"Label: %@\n",object[@"labl"]]];
}
[string appendString:[NSString stringWithFormat:@"Created at: %@\n",object[@"cdat"]]];
if(object[@"agrp"] != nil)
{
[string appendString:[NSString stringWithFormat:@"Belongs to application: %@\n",object[@"agrp"]]];
}
for(NSString* key in @[@"issr",@"subj"])
{
id data = [object objectForKey:key];
@try {
if([data isKindOfClass:[NSData class]]==NO)
{
continue;
}
NSString* stringAscii = [[NSString alloc] initWithData:data encoding:NSASCIIStringEncoding];
NSCharacterSet* alphaNumeric = [NSCharacterSet characterSetWithCharactersInString:@"abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ01234567890.@"];
NSCharacterSet *doNotWant = [alphaNumeric invertedSet];
NSString* cleanedUpString = [[stringAscii componentsSeparatedByCharactersInSet: doNotWant] componentsJoinedByString: @" "];
if(cleanedUpString.length>0)
{
DLog(@" %@ Cleaned up: %@",key,cleanedUpString);
[string appendString:[NSString stringWithFormat:@" %@ Cleaned up: %@",key,cleanedUpString]];
}
}
@catch (NSException *exception) {
}
@finally {
}
}
// [string appendString:[NSString stringWithFormat:@"Complete description:(%@)\n", [object description]]];
return string;
}