So you want to transform your object to minimum enclosing circle,
Like in the below image transform reed rectangle to green circle. That is transform bounding rectangle to bounding circle.
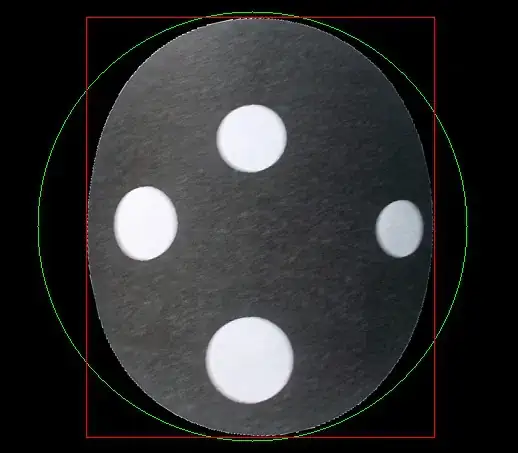
So do the following,
Mat src=imread("src.png");
Mat thr;
cvtColor(src,thr,CV_BGR2GRAY);
threshold( thr, thr, 20, 255,CV_THRESH_BINARY_INV );
bitwise_not(thr,thr);
vector< vector <Point> > contours; // Vector for storing contour
vector< Vec4i > hierarchy;
Mat dst(src.rows,src.cols,CV_8UC1,Scalar::all(0)); //create destination image
findContours( thr.clone(), contours, hierarchy,CV_RETR_EXTERNAL, CV_CHAIN_APPROX_SIMPLE ); // Find the contours in the image
drawContours( dst,contours, 0, Scalar(255,255,255),CV_FILLED, 8, hierarchy );
Rect R=boundingRect(contours[0]);
Point2f center;
float radius;
minEnclosingCircle( (Mat)contours[0], center, radius);`
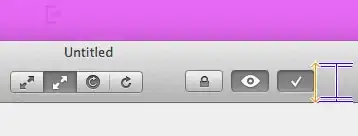
Here you need calculate transformation matrix from bounding box and circle like
std::vector<Point2f> src_pts;
std::vector<Point2f> dst_pts;
src_pts.push_back(Point(R.x,R.y));
src_pts.push_back(Point(R.x+R.width,R.y));
src_pts.push_back(Point(R.x,R.y+R.height));
src_pts.push_back(Point(R.x+R.width,R.y+R.height));
dst_pts.push_back(Point2f(center.x-radius,center.y-radius));
dst_pts.push_back(Point2f(center.x+radius,center.y-radius));
dst_pts.push_back(Point2f(center.x-radius,center.y+radius));
dst_pts.push_back(Point2f(center.x+radius,center.y+radius));
Mat transmtx = getPerspectiveTransform(src_pts,dst_pts);
and then apply perspective transform,
Mat transformed = Mat::zeros(src.rows, src.cols, CV_8UC3);
warpPerspective(src, transformed, transmtx, src.size());
imshow("transformed", transformed);
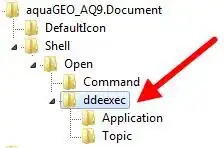