When an error occurs, program execution stops and output is overwritten with an abbreviated version of the error (which provides no useful information).
For example, running the buggy code from here will only show you this:
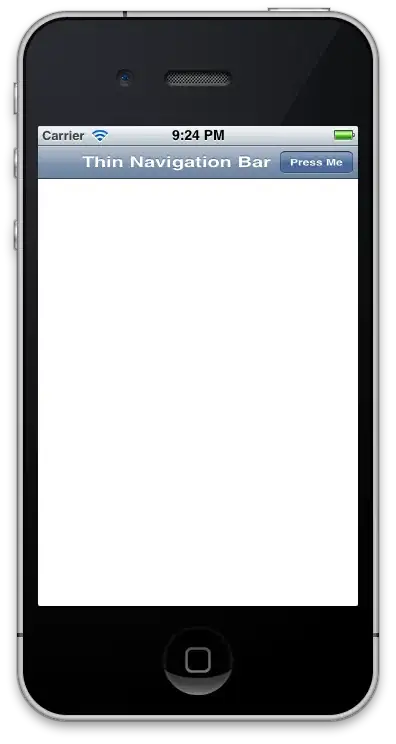
You need to catch all errors to prevent that from happening.
Use try: ... except
:
try:
l = [[1, 2, 3], [4, 5, 6], [7], [8, 9]]
reduce(lambda x, y: x.extend(y), l)
except Exception as error:
print(repr(error))
You will get this printed out:
AttributeError("'NoneType' object has no attribute 'extend'",)
If you want more information, you can just tweak the code:
from sys import exc_info
from traceback import extract_tb
try:
l = [[1, 2, 3], [4, 5, 6], [7], [8, 9]]
reduce(lambda x, y: x.extend(y), l)
except Exception as error:
print(repr(error))
print("Line number: ", extract_tb(exc_info()[2])[0][1])
Prints:
AttributeError("'NoneType' object has no attribute 'extend'",)
('Line number: ', 5)