If I understand you right, your blobs can be regarded as cv::Rect(StartingX,StartingY, BlobWidth, BlobHeight)
in opencv c++
, checking whether such Rects overlap is easy: rectA & rectB = rectOverlap
where rectOverlap is the rect area covered by BOTH rects, so if rectOverlap has .width > 0
and c.height > 0
then the blobs/rects overlap.
here is sample code which creates some rectangles and computes whether they overlap or not:
int main()
{
cv::Mat sample = cv::Mat(512,512,CV_8UC3, cv::Scalar(0,0,0));
// create sample rectangles: positionsX, positionY, width, height
cv::Rect rectA(100,50,50,200);
cv::Rect rectB(50,100,200,50);
cv::Rect rectC(400,50,100,100);
// draw in different colors:
cv::rectangle(sample, rectA, cv::Scalar(0,0,255));
cv::rectangle(sample, rectB, cv::Scalar(255,0,0));
cv::rectangle(sample, rectC, cv::Scalar(0,255,0));
// create output
cv::Mat result = cv::Mat(512,512,CV_8UC3, cv::Scalar(0,0,0));
// compute overlap with overloaded & operator for cv::Rect
cv::Rect overlapAB = rectA & rectB;
cv::Rect overlapAC = rectA & rectC;
// test for overlap and draw or terminal output
if(overlapAB.width && overlapAB.height) cv::rectangle(result, overlapAB, cv::Scalar(255,0,255), -1);
else std::cout << " no overlap between rectA and rectB" << std::endl;
if(overlapAC.width && overlapAC.height) cv::rectangle(result, overlapAC, cv::Scalar(0,255,255), -1);
else std::cout << " no overlap between rectA and rectC" << std::endl;
cv::imshow("rects", sample);
cv::imshow("overlap", result);
cv::imwrite("RectOverlapInput.png", sample);
cv::imwrite("RectOverlapOutput.png", result);
cv::waitKey(-1);
}
and here are input and output. you can see the detected overlap of the blue and red rectangles is the pink rect.
input:
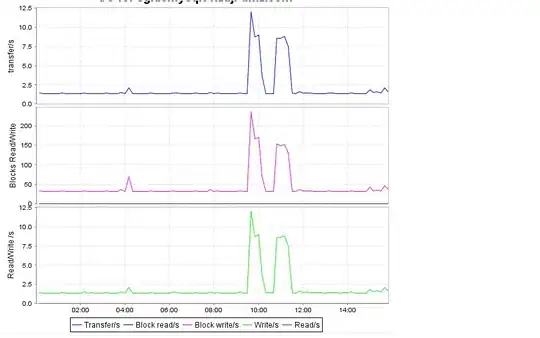
output: no overlap between rectA and rectC
and this image:
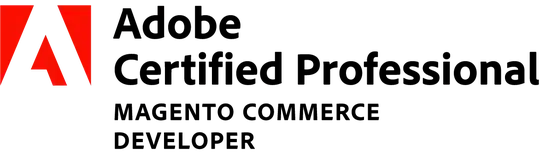