tl;dr
What's the better way to don't get these deprecated alerts?
Do not use that terrible class, java.util.Date
. Use its replacement, Instant
.
myJavaUtilDate.toInstant().plusSeconds( 30 )
java.time
The modern approach uses the java.time classes defined in JSR 310 that supplanted the terrible legacy date-time classes such as Calendar
and Date
.
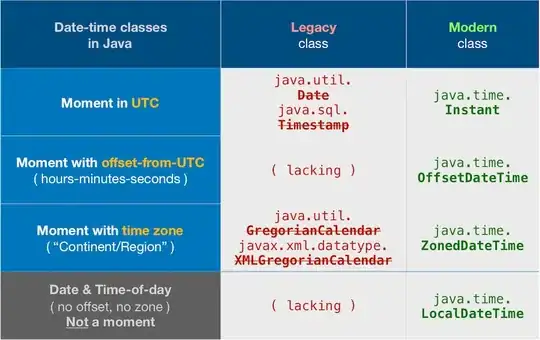
Start of day
Date startTime = dayStart(dateSelected);
If you want the first moment of the day, you need a LocalDate
(date) and a ZoneId
(time zone).
ZoneId z = ZoneId.of( "Africa/Tunis" ) ;
LocalDate localDate = LocalDate.now( z ) ; // Get the current date as seen in a particular time zone.
Get the start of that date. Specify a time zone to get a ZonedDateTime
. Always let java.time determine the first moment of the day. Some dates in some zones do not start at 00:00:00. They might start at another time such as 01:00:00.
ZonedDateTime zdt = localDate.atStartOfDay( z ) ;
Date-time math
You can perform date-time math by calling the plus…
& minus…
methods.
Specify a span-of-time not attached to the timeline using either Duration
or Period
classes.
Duration d = Duration.ofSeconds( 30 ) ;
Add.
ZonedDateTime zdtLater = zdt.plus( d ) ;
Or combine those 2 lines into 1.
ZonedDateTime zdtLater = zdt.plusSeconds( 30 ) ;
Converting
If you need to interoperate with old code not yet updated to java.time, call new conversion methods added to the old classes.
The java.util.Date
legacy class represents a moment in UTC with a resolution of milliseconds. Its replacement is java.time.Instant
, also a moment in UTC but with a much finer resolution of nanoseconds.
You can extract a Instant
from a ZonedDateTime
, effectively adjusting from some time zone to UTC. Same moment, same point on the timeline, different wall-clock time.
Instant instant = zdtLater.toInstant() ;
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.