Here is how you can "transform" an image into a trapezoid shape. The technique is well-known slicing the image line for line and drawing the line scaled a little by little.
This function allows you set amount (%) of trapezoid shape and handles scaled image as well:
function drawTrapezoid(ctx, img, x, y, w, h, factor) {
var startPoint = x + w * 0.5 * (factor*0.01), // calculate top x
xi, yi, scale = img.height / h, // used for interpolation/scale
startLine = y, // used for interpolation
endLine = y + h; // abs. end line (y)
for(; y < endLine; y++) {
// get x position based on line (y)
xi = interpolate(startPoint, y, x, endLine, (y - startLine) / h);
// get scaled y position for source image
yi = (y * scale + 0.5)|0;
// draw the slice
ctx.drawImage(img, 0, yi, img.width, 1, // source line
xi.x, y, w - xi.x * 2, 1); // output line
}
// sub-function doing the interpolation
function interpolate(x1, y1, x2, y2, t) {
return {
x: x1 + (x2 - x1) * t,
y: y1 + (y2 - y1) * t
};
}
}
FIDDLE
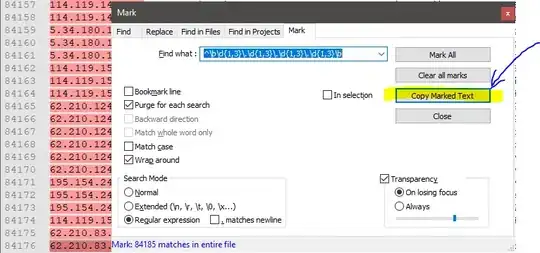
Hope this helps!