There is a useful pattern for doing this sort of thing in regex:
exclusion_context1|exclusion_context2|...|(stuff_you_want)
Where you can specify as many exclusion contexts as you want, and at the end capture the stuff you do want inside a capturing group. I could explain further but really I'll just link you to this answer which goes into great depth about the above pattern.
So, then:
\[.*?\]|(andres)
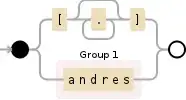
Debuggex Demo
Where our exclusion context lazily matches anything inside brackets, and otherwise we capture all the andres
outside of that context.
Since I just noticed you wanted the positions of the matches, it might look something like this in python:
for m in re.finditer(r'\[.*?\]|(andres)', s):
if m.group(1):
print('{}: {}'.format(m.start(),m.group()))
0: andres
23: andres
39: andres
47: andres