The answer is yes, you get a repeatable sequence, if you always use the same implementation and the same seed, though it might be ill-advised due to possibly poor quality of rand()
.
Better use the C++ random number framework in <random>
though. It not only allows reproducible sequences across implementations, it also supplies all you need to reliably get the distribution you really want.
Now to the details:
The requirements on rand
are:
- Generates pseudo-random numbers.
- Range is 0 to
RAND_MAX
(minimum of 32767).
- The seed set by
srand()
determines the sequence of pseudo-random numbers returned.
There is no requirement on what PRNG is implemented, so every implementation can have its own, though Linear Congrueantial Generators are a favorite.
A conforming (though arguably useless) implementation is presented in this dilbert strip:
http://dilbert.com/strips/comic/2001-10-25/
Or for those who like XKCD (It's a perfect drop-in for any C or C++ library ;-)):
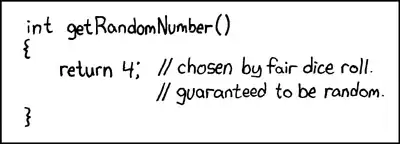
For completeness, the standard quotes:
7.22.2.1 The rand function
The rand function computes a sequence of pseudo-random integers in the range 0 to
RAND_MAX.
[...]
The value of the RAND_MAX macro shall be at least 32767.
7.22.2.2 The srand function
The srand function uses the argument as a seed for a new sequence of pseudo-random
numbers to be returned by subsequent calls to rand. If srand is then called with the
same seed value, the sequence of pseudo-random numbers shall be repeated. If rand is
called before any calls to srand have been made, the same sequence shall be generated
as when srand is first called with a seed value of 1.