There are essentially two ways to do so.
tableHeaderView
The first way involves the tableHeaderView
property of the UITableView
instance you have. You can simply add the UITableView
with the constraints/frame/autoresizingMask that allows you to put it full-screen. Done that, you simply do (i.e. in your viewDidLoad
):
UIView *headerView = [UIView new];
// Here I am supposing that you have a 200pt high view and a `self.tableView` UITableView
headerView.frame = CGRectMake(0, 0, self.tableView.frame.size.width, 200.0);
UIImageView *fixedImageView = [UIImageView new];
// configure your imageView..
[headerView addSubview:fixedImageView];
// configure labels as you want and add them to headerView as subviews
// Now set `UITableView` headerView
self.tableView.tableHeaderView = headerView;
If you want to use AutoLayout for your tableHeaderView, I suggest you to take a look at this question
Dynamic scrollView
Another way to do this is to to create an UIScrollView
, put everything inside, and let it scroll. The downside of this method is that if you are using floating section headers for your UITableView
, they will not float due to the fact that the tableView
is going to stay fixed, while the parent scrollView
is going to scroll.
On the other side, this approach is more AutoLayout friendly due to the fact you can use constraints easily.
To do so, you start adding an UIScrollView
to your view, and placing all your other views inside it.
Be sure to add a Vertical Spacing constraint between the first view inside your scrollView
(I suppose the UIImageView
) and the scrollView
top, and between the last view (I suppose the UITableView
) and the scrollView
bottom, to avoid an ambiguous content size.
You should have something like that (I omitted the labels for the sake of brevity):
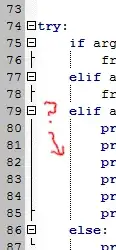
Note that every view is inside a parent UIScrollView
After that, add an Height constraint to the tableView
, and add an IBOutlet
to your view controller subclass, i.e. like this:
@property (weak, nonatomic) IBOutlet NSLayoutConstraint *tableViewHeightConstraint;
Now you only need to configure this constraint to reflect the tableView
natural height, given by its rows, etc. To do so, you simply calculate the height in this way:
// Resize TableView
CGFloat height = self.tableView.contentSize.height;
self.tableViewHeightConstraint.constant = height;
Now the tableView will resize, and due to its constraints it will adapt the parent scrollView
contentSize.
Just be sure to refresh this height constraint anytime you reload the UITableView
dataSource.