1). Please download this zip file from drive:
https://drive.google.com/file/d/0B71R0Zw0m1zQM3RVOWNWM2poVHc/edit?usp=sharing
2) unzip the downloaded zip and put all file same like given libs/armeabi folder given below
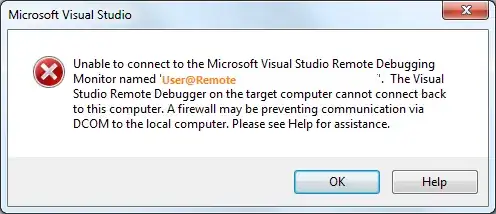
3) use below code in your activty
please import follwing file.
import static com.googlecode.javacv.cpp.opencv_highgui.cvLoadImage;
import com.googlecode.javacv.cpp.avcodec;
import com.googlecode.javacv.cpp.opencv_core.IplImage;
and actual code here
new AsyncTask<Void, Void, Void>() {
ProgressDialog dialog;
protected void onPreExecute() {
dialog = new ProgressDialog(MainActivity.this);
dialog.setMessage("Genrating video, Please wait.........");
dialog.setCancelable(false);
dialog.show();
};
@Override
protected Void doInBackground(Void... arg0) {
File folder = Environment
.getExternalStoragePublicDirectory(Environment.DIRECTORY_DCIM);
String path = folder.getAbsolutePath() + "/Camera";
ArrayList<String> paths = (ArrayList<String>) getListOfFiles(
path, "jpg");
FFmpegFrameRecorder recorder = new FFmpegFrameRecorder(path
+ "/" + "test.mp4", 400, 400);
videoPath = path + "/" + "test.mp4";
try {
//recorder.setVideoCodec(5);
recorder.setVideoCodec(avcodec.AV_CODEC_ID_MPEG4);
//recorder.setFormat("3gp");
recorder.setFormat("mp4");
recorder.setFrameRate(frameRate);
recorder.setVideoBitrate(30);
startTime = System.currentTimeMillis();
recorder.start();
for (int i = 0; i <paths.size(); i++) {
IplImage image = cvLoadImage(path + "/" + paths.get(i));
long t = 3000 * (System.currentTimeMillis() - startTime);
if (t > recorder.getTimestamp()) {
recorder.setTimestamp(t);
recorder.record(image);
}
}
System.out.println("Total Time:- " + recorder.getTimestamp());
recorder.stop();
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
protected void onPostExecute(Void result) {
dialog.dismiss();
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setDataAndType(Uri.parse(videoPath), "video/mp4");
startActivity(intent);
Toast.makeText(MainActivity.this, "Done", Toast.LENGTH_SHORT)
.show();
};
}.execute();
please free to asked if you have any query.