There is no easy, "one-liner" way to do this. However, one way forward isn't so bad. The only thing you need to think about is how to map your time values to colors. Here's one possible way to proceed:
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
N_points = 10
x = np.arange(N_points, dtype=float)
y = x
z = np.random.rand(N_points)
t = x
fig = plt.figure()
ax = fig.gca(projection='3d')
# colors need to be 3-tuples with values between 0-1.
# if you want to use the time values directly, you could do something like
t /= max(t)
for i in range(1, N_points):
ax.plot(x[i-1:i+1], y[i-1:i+1], z[i-1:i+1], c=(t[i-1], 0, 0))
plt.show()
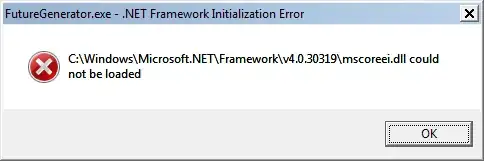
You can play around with that tuple. Having one value with 2 zeros will give you shades of red, green and blue depending on the position of the nonzero argument. Some other possible color choices could be shades of gray
c = (t[i-1], t[i-1], t[i-1])
or instead cycling through a list of predefined colors:
# Don't do: t /= max(t)
from itertools import cycle
colors = cycle('bgrc')
for i in range(1, N_points):
ax.plot(x[i-1:i+1], y[i-1:i+1], z[i-1:i+1], c=colors[t[i-1]])
plt.show()
However, the depends on how you defined your time.