Because of the code -
Protected void btn_Click(object sender, EventArgs e)
{
DataSet ds = new DataSet();
DataTable dt = new DataTable("abc");
dt = getDT(formatDate);
ds.Tables.Add(dt);
GridView2.DataSource = ds.Tables["abc"].DefaultView;
GridView2.DataBind();
}
}
private DataTable getDT(string[] date)
{
DataTable dt = new DataTable();
dt.Columns.Add("RowID", typeof(Int16));
dt.Columns.Add("Date", typeof(DateTime));
for (int i = 0; i < date.Length; i++)
{
dt.Rows.Add(i + 1, date[i]);
}
return dt;
}
Inside the getDT
function you are again creating a new DataTable
which does not have a name. You should either send the table as reference or create it inside the function. Either of the following solution will work -
Protected void btn_Click(object sender, EventArgs e)
{
DataSet ds = new DataSet();
DataTable dt = new DataTable("abc");
dt = getDT(dt, formatDate);
ds.Tables.Add(dt);
GridView2.DataSource = ds.Tables["abc"].DefaultView;
GridView2.DataBind();
}
}
private DataTable getDT(DataTable dt, string[] date)
{
//DataTable dt = new DataTable();
dt.Columns.Add("RowID", typeof(Int16));
dt.Columns.Add("Date", typeof(DateTime));
for (int i = 0; i < date.Length; i++)
{
dt.Rows.Add(i + 1, date[i]);
}
return dt;
}
OR,
Protected void btn_Click(object sender, EventArgs e)
{
DataSet ds = new DataSet();
DataTable dt = getDT(formatDate);
ds.Tables.Add(dt);
GridView2.DataSource = ds.Tables["abc"].DefaultView;
GridView2.DataBind();
}
}
private DataTable getDT(string[] date)
{
DataTable dt = new DataTable("abc");
dt.Columns.Add("RowID", typeof(Int16));
dt.Columns.Add("Date", typeof(DateTime));
for (int i = 0; i < date.Length; i++)
{
dt.Rows.Add(i + 1, date[i]);
}
return dt;
}
EDIT: Only for HassanNisar
@HassanNisar .. this is the debugger output -
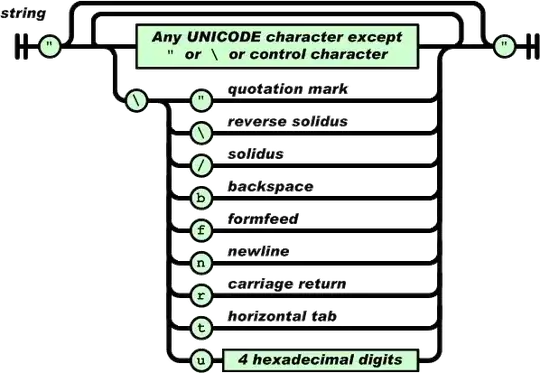