I've come up with a pure-JavaScript solution which combines Nick R's and alhoseany's answers together, whilst adding in a few extra bits of functionality to detect length and specify the number of characters required either side of the ellipsis.
With this method you do not need to know the number of characters that can fit in your container, it's all done automatically and can also be triggered when the window resizes.
Resize the result frame to see the results in action.
Result
This:

...becomes this:
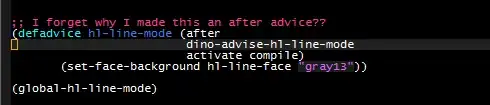
...or this:
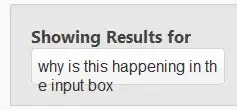
...or this!
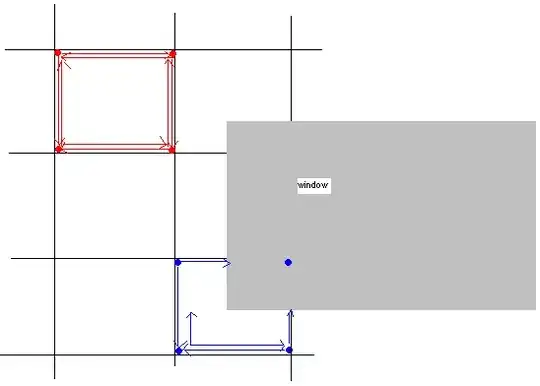
The Code
CSS
p {
border: 1px dashed #000;
position: relative;
display: block;
width: 50%;
}
p:before {
content:attr(data-shortened);
color: #000;
display: block;
position: absolute;
top: 0;
left: 0;
width: 100%;
}
JavaScript
function shortenContent(content, chars) {
/* chars here determines how many characters
* we want on either side of the elipsis. */
var chars = chars || 3; // Default is 3
if (!content && !content.length)
return;
/* Loop through each content element and
* shorten where necessary. */
for (i = 0; i < content.length; i++) {
var el = content[i],
elementWidth = el.offsetWidth,
textWidth = el.scrollWidth;
/* If our element's width is less than
* its content's width, we need to shorten. */
if (elementWidth < textWidth) {
var text = el.innerText;
/* Set the data attribute for the CSS to use. */
el.setAttribute(
'data-shortened', text.slice(0,chars) +'...'+ text.slice(-chars)
);
el.style.color = 'rgba(0, 0, 0, 0)';
}
/* Otherwise, ensure non-shortened text is visible. */
else {
el.setAttribute('data-shortened', '');
el.style.color = null;
}
}
}
How do I use it?
To use the above function, you simply need to pass in a collection of elements into the shortenContent
function:
// Get the content we wish to shorten
var content = document.querySelectorAll('div p');
/* Trigger the function initially. */
shortenContent(content);
abcdefghijklmnopqrstuvwxyz => abc...xyz
Specifying a different number of characters
If you want a different number of characters to appear before and after the ellipsis (e.g. abcd...wxyz
instead of abc...xyz
), you can pass in a number as a second argument into the shortenContent
function:
/* Trigger the function initially. */
shortenContent(content, 4);
abcdefghijklmnopqrstuvwxyz => abcd...wxyz
Window Resize example
This will fire the shortenContent
function whenever the window (the JSFiddle result pane, in this case) changes size.
/* Fire the shorten function when the window resizes. */
window.onresize = function(event) {
shortenContent(content);
};