Two ways: (1) not precise solution as text alignment should be coded by hands but:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button view1 = new Button(this);
RelativeLayout layout1 = (RelativeLayout) findViewById(R.id.layout1);
layout1.setBackground(getResources().getDrawable(R.drawable.choose_background));
layout1.addView(view1);
Bitmap mainImage = Bitmap.createBitmap(500, 500, Bitmap.Config.ARGB_8888);
{
//creating image is not necessary, more important is ability to grab the bitmap.
Canvas canvas = new Canvas(mainImage);
canvas.drawColor(Color.WHITE);
view1.setBackground(new BitmapDrawable(getResources(), mainImage));
}
{
Canvas canvas = new Canvas(mainImage);
//most interesting part:
Paint eraserPaint = new Paint();
eraserPaint.setAlpha(0); //this
eraserPaint.setTextSize(200);
eraserPaint.setXfermode(new PorterDuffXfermode(PorterDuff.Mode.DST_IN)); //and this
canvas.drawText("some", 10, 150, eraserPaint);
}
}
the idea of eraserPaint is from here Erase bitmap parts using PorterDuff mode and here Android how to apply mask on ImageView?
in activity_main.xml everything is default:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/layout1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.runup.myapplication2.app.MainActivity">
and the result is:
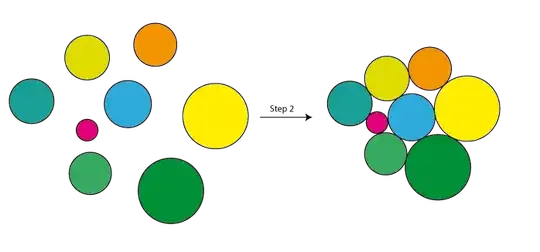
(2) Second way is to add this behavior to class in order to use it in xml.
https://github.com/togramago/ClearTextViewProject - library project with a sample. You may add as a library or copy only ClearTextView class to your project (as it is an extended TextView without xml resources). works on configuration change and dynamical text and/or background changes.
The result of the sample in portrait:
and in landscape: 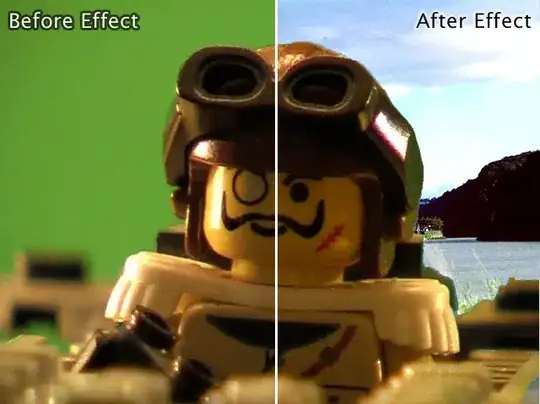