Copying my own answer from Is there any way to access certificate information from a Chrome Extension
2018 answer: yes, in Firefox 62
You'll need to make a WebExtension, which is also called a browser extension.
See accessing security information on MDN
You can also check out the docs for:
You'll need Firefox 62.
Here's a working background.js
var log = console.log.bind(console)
log(`\n\nTLS browser extension loaded`)
// https://developer.chrome.com/extensions/match_patterns
var ALL_SITES = { urls: ['<all_urls>'] }
// Mozilla doesn't use tlsInfo in extraInfoSpec
var extraInfoSpec = ['blocking'];
// https://developer.mozilla.org/en-US/Add-ons/WebExtensions/API/webRequest/onHeadersReceived
browser.webRequest.onHeadersReceived.addListener(async function(details){
log(`\n\nGot a request for ${details.url} with ID ${details.requestId}`)
// Yeah this is a String, even though the content is a Number
var requestId = details.requestId
var securityInfo = await browser.webRequest.getSecurityInfo(requestId, {
certificateChain: true,
rawDER: false
});
log(`securityInfo: ${JSON.stringify(securityInfo, null, 2)}`)
}, ALL_SITES, extraInfoSpec)
log('Added listener')
manifest.json
:
{
"manifest_version": 2,
"name": "Test extension",
"version": "1.0",
"description": "Test extension.",
"icons": {
"48": "icons/border-48.png"
},
"background": {
"scripts": ["background.js"]
},
"permissions": [
"webRequest",
"webRequestBlocking",
"<all_urls>"
]
}
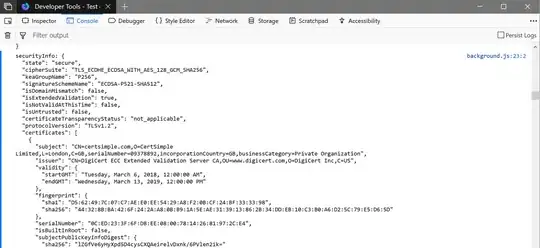
It also may be implemented in Chromium once this code is merged.