You are adding your JTextArea
to your content pane where you should be actually adding your JScrollPane
to the content pane of your JFrame
. You should add the JTextArea
to the content pane of the JScrollPane
. Below is an example of the visible scroll bar in action:
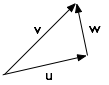
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
public class SimpleScrollBars extends JFrame {
private static final long serialVersionUID = 1L;
private JPanel contentPane;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
SimpleScrollBars frame = new SimpleScrollBars();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public SimpleScrollBars() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
contentPane.setLayout(new BorderLayout(0, 0));
setContentPane(contentPane);
JScrollPane scrollPane = new JScrollPane();
scrollPane.setVerticalScrollBarPolicy(JScrollPane.VERTICAL_SCROLLBAR_ALWAYS);
contentPane.add(scrollPane, BorderLayout.CENTER);
JTextArea textArea = new JTextArea(5, 15);
scrollPane.setViewportView(textArea);
pack();
}
}