Here's the problem - you're usually wrong to keep the OBJECTID of the company, in Post.
You should simply keep an object there .. the column in Post can actually be a pointer to an "Company".
Here's two examples...
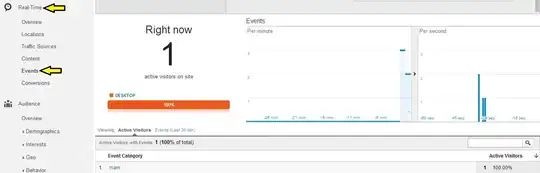
Notice this "Post" table literally has a USER as a column, i called it "author:".

Here a "Comment" table has an "author" column which is literally a "User" and the "regardingPost" is again a pointer column, it's literally a Post.
(Note that in the Parse dash, it shows the ID, of, the object you are pointing to - very handy.)
This is a basic of using Parse. In the first instance, you need to go back and rework your tables so that you have, in your example, your table "Post" simply contains a pointer to company.
{Footnote - in some obscure cases, in Parse, you do need to keep the 'actual' objectID rather than the item itself, the pointer. That is not relevant here.}
So, first fix that. Now you ask, how to easily get that "company image" when you get a Post.
This is one of the central amazing things about Parse - it's incredibly easy. It's what the "include" concept comes in. Your query would look something like this...
PFQuery *QQ = [PFQuery queryWithClassName:@"Posts"];
[QQ whereKey:@"subject" equalTo:"Farming"];
QQ.limit = 1000;
[QQ includeKey:@"author"];
QQ.cachePolicy = kPFCachePolicyCacheThenNetwork;
[QQ orderByDescending:@"createdAt"];
notice the "includeKey".
Then, let's say you have your "Posts". You're going through a loop and each post is "onePost".
So of course you can do this...
topic = [aPost objectForKey:@"topic"];
city = [aPost objectForKey:@"cityCode"];
state = [aPost objectForKey:@"state"];
and so on. to get the whole company object, you simply do this...
PFObject *thatCompany = [aPost objectForKey:@"company"];
and that's why we all use Parse!
then of course you can get anything from thatCompany, like the image
yourImage = [thatCompany objectForKey:@"companyImage"];
Don't forget to do this, you would have converted your table to use an actual pointer column as I explain above.
Note that pointer columns (which are what you "always do" in parse, they are ubiquitous -- most of your columns will be pointer columns) are well explained simply on Parse.com, click to documentation and on the left click iOS.
I hope this helps because it took a long time to write :) Cheers
Just FYI this may help you. Here's the correct way to load images in to a UIImageView.
@implementation UIImageView (Hexaface)
-(void)loadAvatarImage:(PFUser *)whom
// literally, for this UIImageView, set the user avatar image
{
if ( whom == nil ) return;
PFFile *parseImageFile = [whom objectForKey:@"yourImageColumn"];
__weak UIImageView *mySelf = self;
if ( parseImageFile != nil )
{
[parseImageFile getDataInBackgroundWithBlock:^(NSData *result, NSError *error)
{
if ( error != nil ) { return; }
UIImage *image = [UIImage imageWithData:result];
mySelf.image = image;
}];
}
else
{
NSLog(@"catastrophe, loadAvatarImage found no image ...............");
}
}
If you are a beginner programmer and you are not using categories, either (a) just adopt the code and make it a normal call in your class. Or, I strongly recommend this is the perfect opportunity to learn about categories. Remember that in iOS you use categories constantly, always (much if nor most of your code will be categories).
Note I will tip you an incredibly useful tip for working with iOS and Parse. There is an amazingly useful library called...
DLImageLoader
which you more or less have to use when working with images in iOS particularly with Parse. DLImageLoader is your solution.
https://stackoverflow.com/a/19115912/294884
full working example there. the library is completely free, grab the two files and use it. Hope it helps.