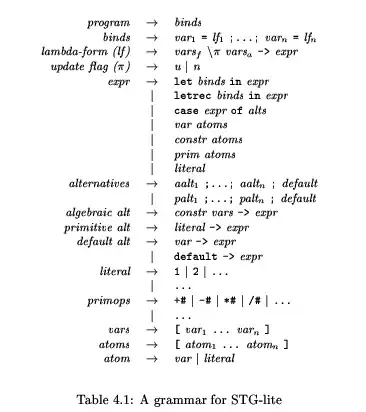
Try the below example:
import java.awt.Color;
import java.awt.EventQueue;
import java.awt.FlowLayout;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.Insets;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.DefaultCellEditor;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.table.DefaultTableCellRenderer;
import javax.swing.table.DefaultTableModel;
import javax.swing.table.TableColumn;
public class CreateTargetButton {
private JPanel panel;
private JTable table;
private JScrollPane scrollPane;
DefaultTableModel model;
DefaultTableModel dtm;
private JFrame CreateTarget_frame = new JFrame();
@SuppressWarnings("rawtypes")
private JComboBox datatype_comboBox = new JComboBox();
//private boolean blob_clob_ind = false;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
CreateTargetButton frame = new CreateTargetButton();
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public CreateTargetButton(){
CreateTarget_frame.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
panel = new JPanel();
panel.setBackground(new Color(214,227,241));
CreateTarget_frame.setContentPane(panel);
GridBagLayout gbl_panel = new GridBagLayout();
gbl_panel.columnWidths = new int[]{0, 0};
gbl_panel.rowHeights = new int[]{0, 0};
gbl_panel.columnWeights = new double[]{1.0, Double.MIN_VALUE};
gbl_panel.rowWeights = new double[]{1.0, Double.MIN_VALUE};
panel.setLayout(gbl_panel);
JPanel panel_2 = new JPanel();
GridBagConstraints gbc_panel_2 = new GridBagConstraints();
gbc_panel_2.fill = GridBagConstraints.BOTH;
gbc_panel_2.insets = new Insets(2, 5, 5, 5);
gbc_panel_2.weighty = 95;
gbc_panel_2.gridx = 0;
gbc_panel_2.gridy = 1;
panel.add(panel_2, gbc_panel_2);
GridBagLayout gbl_panel_2 = new GridBagLayout();
gbl_panel_2.columnWidths = new int[]{0, 0};
gbl_panel_2.rowHeights = new int[]{0, 0};
gbl_panel_2.columnWeights = new double[]{1.0, Double.MIN_VALUE};
gbl_panel_2.rowWeights = new double[]{1.0, Double.MIN_VALUE};
panel_2.setLayout(gbl_panel_2);
scrollPane = new JScrollPane();
GridBagConstraints gbc_scrollPane = new GridBagConstraints();
gbc_scrollPane.fill = GridBagConstraints.BOTH;
gbc_scrollPane.gridx = 0;
gbc_scrollPane.gridy = 0;
panel_2.add(scrollPane, gbc_scrollPane);
JPanel panel_3 = new JPanel();
panel_3.setBackground(new Color(214,227,241));
GridBagConstraints gbc_panel_3 = new GridBagConstraints();
gbc_panel_3.fill = GridBagConstraints.BOTH;
gbc_panel_3.weighty = 5;
gbc_panel_3.gridx = 0;
gbc_panel_3.gridy = 2;
panel.add(panel_3, gbc_panel_3);
GridBagLayout gbl_panel_3 = new GridBagLayout();
gbl_panel_3.columnWidths = new int[]{0, 0};
gbl_panel_3.rowHeights = new int[]{0, 0};
gbl_panel_3.columnWeights = new double[]{1.0, Double.MIN_VALUE};
gbl_panel_3.rowWeights = new double[]{1.0, Double.MIN_VALUE};
panel_3.setLayout(gbl_panel_3);
JPanel SUB_panel_3_1 = new JPanel();
SUB_panel_3_1.setBackground(new Color(214,227,241));
GridBagConstraints gbc_SUB_panel_3_1 = new GridBagConstraints();
gbc_SUB_panel_3_1.fill = GridBagConstraints.BOTH;
gbc_SUB_panel_3_1.weightx = 5;
gbc_SUB_panel_3_1.gridx = 0;
gbc_SUB_panel_3_1.gridy = 0;
panel_3.add(SUB_panel_3_1, gbc_SUB_panel_3_1);
GridBagLayout gbl_SUB_panel_3_1 = new GridBagLayout();
gbl_SUB_panel_3_1.columnWidths = new int[]{0, 0, 0};
gbl_SUB_panel_3_1.rowHeights = new int[]{0, 0};
gbl_SUB_panel_3_1.columnWeights = new double[]{0.0, 0.0, Double.MIN_VALUE};
gbl_SUB_panel_3_1.rowWeights = new double[]{0.0, Double.MIN_VALUE};
SUB_panel_3_1.setLayout(gbl_SUB_panel_3_1);
JButton button_add_column = new JButton("+");
GridBagConstraints gbc_button = new GridBagConstraints();
gbc_button.insets = new Insets(0, 10, 0, 5);
gbc_button.gridx = 0;
gbc_button.gridy = 0;
SUB_panel_3_1.add(button_add_column, gbc_button);
JButton button_del_column = new JButton("-");
GridBagConstraints gbc_button_1 = new GridBagConstraints();
gbc_button_1.gridx = 1;
gbc_button_1.gridy = 0;
SUB_panel_3_1.add(button_del_column, gbc_button_1);
JPanel SUB_panel_3_3 = new JPanel();
SUB_panel_3_3.setBackground(new Color(214,227,241));
GridBagConstraints gbc_SUB_panel_3_3 = new GridBagConstraints();
gbc_SUB_panel_3_3.gridwidth = 2;
gbc_SUB_panel_3_3.fill = GridBagConstraints.BOTH;
gbc_SUB_panel_3_3.weightx = 90;
gbc_SUB_panel_3_3.gridx = 1;
gbc_SUB_panel_3_3.gridy = 0;
panel_3.add(SUB_panel_3_3, gbc_SUB_panel_3_3);
SUB_panel_3_3.setLayout(new FlowLayout(FlowLayout.RIGHT, 5, 5));
button_add_column.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
dtm.addRow(new Object[]{new Boolean(false),"", "",
"", new Integer(5)});
}
});
button_del_column.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
for(int i=0; i<dtm.getRowCount();i++)
{
String temp = dtm.getDataVector().elementAt(i).toString();
temp = temp.substring(1,5);
if(temp.equals("true"))
{
dtm.removeRow(i);
i--;
}
}
}
});
tablecall();
CreateTarget_frame.pack();
CreateTarget_frame.setVisible(true);
}
private void tablecall()
{
String[] columnNames = {"",
"Column Name",
"Data Type",
"Size"};
Object[][] data = {
{new Boolean(false),"", "",
"", new Integer(5)}};
model = new DefaultTableModel(data, columnNames)
// { public boolean isCellEditable(int row, int column) {
// if(blob_clob_ind)
// return false;
// else
// return true;
// }
// }
;
table = new JTable(model){
private static final long serialVersionUID = 1L;
@SuppressWarnings({ "unchecked", "rawtypes" })
public Class getColumnClass(int column) {
switch (column) {
case 0:
return Boolean.class;
case 1:
return String.class;
case 2:
return String.class;
case 3:
return Integer.class;
default:
return Boolean.class;
}
}
};
dtm = (DefaultTableModel) table.getModel();
TableColumn column = null;
for (int i = 0; i < 3; i++) {
column = table.getColumnModel().getColumn(i);
if (i == 0) {
column.setMaxWidth(10);
}
}
setUpSportColumn(table, table.getColumnModel().getColumn(2));
scrollPane.getViewport().add(table);
}
@SuppressWarnings({ "unchecked" })
public void setUpSportColumn(JTable table,
TableColumn sportColumn) {
//Set up the editor for the sport cells.
//datatype_comboBox.addItem("BLOB");
//datatype_comboBox.addItem("CLOB");
datatype_comboBox.addItem("Integer");
datatype_comboBox.addItem("Varchar2");
//datatype_comboBox.addItem("Date");
sportColumn.setCellEditor(new DefaultCellEditor(datatype_comboBox));
//Set up tool tips for the sport cells.
DefaultTableCellRenderer renderer =
new DefaultTableCellRenderer();
renderer.setToolTipText("Click for combo box");
sportColumn.setCellRenderer(renderer);
/*
datatype_comboBox.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
if(datatype_comboBox.getSelectedIndex() == 0 ||datatype_comboBox.getSelectedIndex() == 1)
}
});
*/
}
}