This question is a variation on the following questions by the same OP:
The previous versions of this were very unclear. Based on the comments on the previous questions, the OP wants to take the Outline tree using the SimpleBookmark
class, change the Destinations similar to the way he changed the destinations of annotations (as shown in C# - Set inherit zoom action for all the bookmarks in the PDF file) and then persist these changes.
When using SimpleBookmark
, you get the outlines in a form that is similar to this XML-file:
<Bookmark>
<Title Action="GoTo" Style="bold" Page="1 FitH 572">Akira</Title>
<Bookmark>
You can change this XML file (or in the case of the OP the contents of the Dictionary<string, object>
obtained from SimpleBookmark
), for instance, you could change 1 Fith 572
into 1 Fith 580
. This is outside the scope of iText: it's a matter of changing XML or changing Strings in a Dictionary.
Once you've made the change, you need to persist the change in the PDF. For instance, you have a bookmarks
object:
List<Dictionary<String, Object>> bookmarks;
and all the changes are reflected in this bookmarks
object.
Now you can use PdfStamper
's setOutline()
method (Java) to change the outlines of the document. In C#, it would be something like this:
stamper.Outlines = bookmarks;
This is only one way to approach it. Obviously, it's also possible to walk through the outline objects and change the destinations at the lowest PDF level.
If you want an example, see the ChangeBookmarks sample:
public void manipulatePdf(String src, String dest) throws IOException, DocumentException {
PdfReader reader = new PdfReader(src);
PdfStamper stamper = new PdfStamper(reader, new FileOutputStream(dest));
List<HashMap<String, Object>> list = SimpleBookmark.getBookmark(reader);
changeList(list);
stamper.setOutlines(list);
stamper.close();
reader.close();
}
public void changeList(List<HashMap<String, Object>> list) {
for (HashMap<String, Object> entry : list) {
for (String key : entry.keySet()) {
if ("Kids".equals(key)) {
Object o = entry.get(key);
changeList((List<HashMap<String, Object>>)o);
}
else if ("Page".equals(key)) {
String dest = (String)entry.get(key);
entry.put("Page", dest.replaceAll("Fit", "FitV 60"));
}
}
}
}
As already explained, you get all the bookmarks as a List<HashMap<String, Object>>
. You write a recursive method that goes through the outline tree ("Kids"
) and changes all the values of the Page
entry. In my case, the bookmarks in bookmarks.pdf are all of type "Fit". I replace "Fit" with "FitV 60". Take a look at changed_bookmarks.pdf to see the difference:
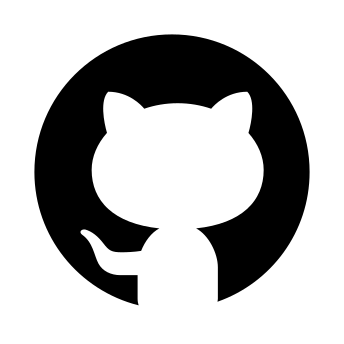
Suppose that you want an XYZ destination where you inherit the zoom factor, you can take do something that is similar to what you did in a previous question you posted: How to set zoom level to pdf using iTextSharp?
Instead of replacing "Fit" with "FitV 60", you can replace "Fit" by "XYZ 30 100 0". In this case, 30 and 100 are X,Y coordinates. The third number is the zoom level, but if you choose 0, you get "Inherit zoom".