Here is a proposal. But it contains some flaws yet
- Size propagation is fiexed (may be better client or server based sizing)
- No event delegation to underlying
Component
- Performance, because heavyweight panel instances are created frequently inside paint loop
But here is how it can be done. The code is separated into parts.
A value class
Just a simple class to represent the date inside your panels for each cell.
class Value {
public final String text;
public final boolean flag;
public Value(String text, boolean flag) {
this.text = text;
this.flag = flag;
}
}
My individual panel class
The representation can be modelled within a gui editor like google's window builder. This panel makes use of the value and displays it accordingly.
public class MyPanel extends JPanel {
public MyPanel(Value v) {
JLabel lblNewLabel = new JLabel(v.text);
add(lblNewLabel);
JCheckBox chckbxSomeValue = new JCheckBox("some value");
chckbxSomeValue.setSelected(v.flag);
add(chckbxSomeValue);
}
}
A table cell renderer class
Just returns some panel instance showing up the desired values.
import javax.swing.JTable;
import javax.swing.table.TableCellRenderer;
class MyPanelCellRenderer implements TableCellRenderer {
@Override
public Component getTableCellRendererComponent(JTable table, Object value,
boolean isSelected, boolean hasFocus, int row, int column) {
return new MyPanel((Value)value); // maybe performance problem
}
}
A custom table model
import javax.swing.table.DefaultTableModel;
class MyTableModel extends DefaultTableModel {
public MyTableModel() {
super(new Object[][] {
new Object[] { 1, new Value("asdf", true) },
new Object[] { 2, new Value("qwer", false) } },
new String[] {"Id", "MyPanel" });
}
Class[] columnTypes = new Class[] { Integer.class, Value.class };
MyTableModel(Object[][] data, Object[] columnNames) {
super(data, columnNames);
}
public Class getColumnClass(int columnIndex) {
return columnTypes[columnIndex];
}
}
A frame class
import java.awt.BorderLayout;
public class MyFrame {
JFrame frame;
private JTable table;
public MyFrame() {
frame = new JFrame();
frame.setBounds(100, 100, 450, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
table = new JTable();
table.setModel(new MyTableModel());
table.setFillsViewportHeight(true);
table.getColumnModel()
.getColumn(1)
.setCellRenderer(new MyPanelCellRenderer());
table.setRowHeight(40); // static sizing
JScrollPane scrollPane = new JScrollPane();
frame.getContentPane().add(scrollPane, BorderLayout.CENTER);
scrollPane.setViewportView(table);
}
}
Main Function
import java.awt.EventQueue;
public class MyApp {
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
MyFrame window = new MyFrame();
window.frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
}
The final result
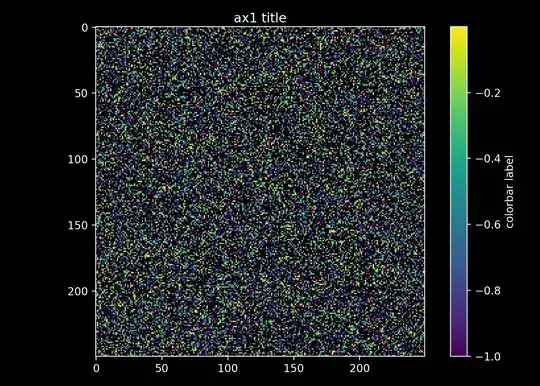
The panel MyPanel
is created using eclipse and google's window builder
