You could start by listing the available font names using something like...
String fonts[]
= GraphicsEnvironment.getLocalGraphicsEnvironment().getAvailableFontFamilyNames();
for (int i = 0; i < fonts.length; i++) {
System.out.println(fonts[i]);
}
This a great little way of checking the font names.
If you did this, you would find that "Comic Sans" is listed as "Comic Sans MS", this means you'll need to use something like new Font("Comic Sans MS", Font.PLAIN, 24)
to create a new font
For example...
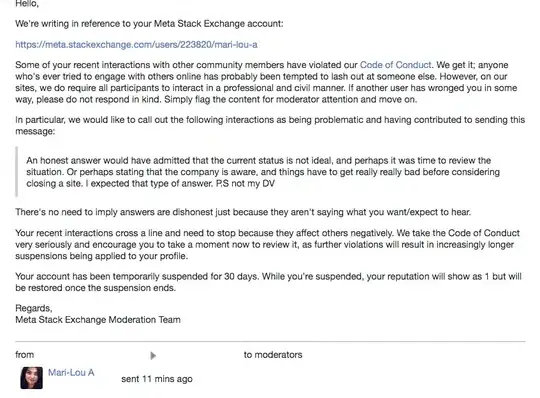
Showing: Comic Sans MS, Calibri, Look and feel default
public class TestPane extends JPanel {
public TestPane() {
setLayout(new GridBagLayout());
JLabel label = new JLabel("Hello");
label.setFont(new Font("Comic Sans MS", Font.PLAIN, 24));
GridBagConstraints gbc = new GridBagConstraints();
gbc.gridwidth = GridBagConstraints.REMAINDER;
add(label, gbc);
label = new JLabel("Hello");
label.setFont(new Font("Calibri", Font.PLAIN, 24));
add(label, gbc);
label = new JLabel("Hello");
Font font = label.getFont();
label.setFont(font.deriveFont(Font.PLAIN, 24f));
add(label, gbc);
}
@Override
public Dimension getPreferredSize() {
return new Dimension(200, 200);
}
}