It does. You may add Enterprise Library 6 into your project via Nuget Here is the sample application.
using System;
using System.Diagnostics;
using Microsoft.Practices.EnterpriseLibrary.Logging;
using Microsoft.Practices.EnterpriseLibrary.Logging.Formatters;
using Microsoft.Practices.EnterpriseLibrary.Logging.TraceListeners;
namespace Practice.Logging
{
internal class Program
{
public static void Main(string[] args)
{
LoggingConfiguration loggingConfiguration = BuildProgrammaticConfig();
var defaultWriter = new LogWriter(loggingConfiguration);
// Check if logging is enabled before creating log entries.
if (defaultWriter.IsLoggingEnabled())
{
defaultWriter.Write("Log entry created using the simplest overload.");
defaultWriter.Write("Log entry with a single category.", "General");
defaultWriter.Write("Log entry with a category, priority, and event ID.", "General", 6, 9001);
defaultWriter.Write("Log entry with a category, priority, event ID, " + "and severity.", "General", 5, 9002, TraceEventType.Warning);
defaultWriter.Write("Log entry with a category, priority, event ID, " + "severity, and title.", "General", 8, 9003, TraceEventType.Warning, "Logging Block Examples");
}
else
{
Console.WriteLine("Logging is disabled in the configuration.");
}
}
private static LoggingConfiguration BuildProgrammaticConfig()
{
// Formatter
var formatter = new TextFormatter();
// Trace Listeners
var eventLog = new EventLog("Application", ".", "StackOverflow #24309323");
var eventLogTraceListener = new FormattedEventLogTraceListener(eventLog, formatter);
// Build Configuration
var config = new LoggingConfiguration();
config.AddLogSource("General", SourceLevels.All, true)
.AddTraceListener(eventLogTraceListener);
config.IsTracingEnabled = true;
return config;
}
}
}
You may find more details in Logging Application Block
To install the extension into the Visual Studio 2013 you may follow the workaround steps below.
- download Microsoft.Practices.EnterpriseLibrary.ConfigConsoleV6.vsix from the link
A VSIX file is a zip file that uses the Open Packaging Convention.
You can rename the .VSIX extension to .ZIP and use any zip browser
(including the Windows File Explorer) to browse its contents.
- extract the file into a folder
- locate the file called extension.vsixmanifest in the folder
- open the file with notepad.exe
- locate
<SupportedProducts>
<VisualStudio Version="11.0">
<Edition>Ultimate</Edition>
<Edition>Premium</Edition>
<Edition>Pro</Edition>
</VisualStudio>
</SupportedProducts>
- and replace it with the part below
<SupportedProducts>
<VisualStudio Version="11.0">
<Edition>Ultimate</Edition>
<Edition>Premium</Edition>
<Edition>Pro</Edition>
</VisualStudio>
<VisualStudio Version="12.0"> <!-- VS2013 -->
<Edition>Ultimate</Edition>
<Edition>Premium</Edition>
<Edition>Pro</Edition>
</VisualStudio>
<VisualStudio Version="14.0"> <!-- VS2015 -->
<Edition>Ultimate</Edition>
<Edition>Premium</Edition>
<Edition>Pro</Edition>
</VisualStudio>
</SupportedProducts>
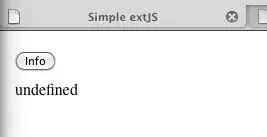
- save the file and exit
- compress folder as a
ZIP
file again
- rename the extension to
VSIX
- double click on it.