My best guess is that setting the width of the image to 200px and leaving the height unspecified is causing the browser to calculate the height of the image. If the height calculates to a nice whole number it isn't an issue. If the height calculates to a decimal it may be the cause of the problem.
In this case the natural dimensions of the image are 275px by 183px.
By changing the width of the image to 200px you are shrinking the image to 72.727272...% of its natural size.
275/200 = 0.727272... Or if you prefer fractions: 275(8/11) = 200
Now running the same equation on the height yields:
183(8/11) = 133.090909...
It looks like, under the normal run of things, the partial pixels are cropped, but during the transition the partial pixels aren't being cropped, and the image is warped slightly to show the partial pixels within the same height.
Cropped down to 133px:
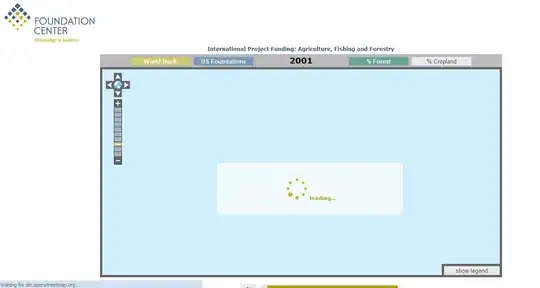
Not cropped and slightly warped:
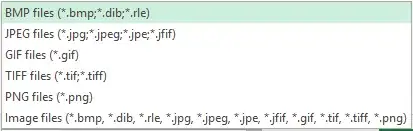
Now that we have a good hypothesis on what's causing the problem, on to the solutions:
You can hard code the height of the image:
Working Example
.link-box .image img {
transition: all .2s ease-out;
width:200px;
height: 133px; /* manually set the height */
}
Or if you would rather not hard code the height, you can also fix the issue with an anti-alias hack, just add a box-shadow.
Working Example
.link-box.large:hover .image img {
opacity: .65;
box-shadow: 0 0 0 #000; /* add a non-visible box-shadow */
}
Or if you're concerned about the cross-browser compatibility of using a box-shadow, you can also use a transparent border:
Working Example
.link-box .image img {
transition: all .2s ease-out;
width:200px;
border: 1px solid transparent; /* add transparent border */
}