The reason that val has changed is because in Java, "objects" are essentially pointers that point to that object in memory. In your code, you define a NEW object (and a pointer called v1 that points to it) for a Value. You then specify that you want the value to be 5. When you go to create you second value object, you instead are creating another POINTER, and having that pointer ALSO point at your first object. Therefore when you change the value that your pointer is pointing at, you change the object itself. Since both pointers point at this object, it appears that both are the same.
What you should do instead is:
Value v1 = new Value();
v1.val = 5;
Value v2 = new Value();
v2.val = v1.val;
v2.val = 6;// how val changes from 5 to 6
This creates a pointer pointing to a NEW object of the type Value, then sets the value of that object to the other objects value. This way when you reference them, they remain separate as they point to different spots in memory.
Here is an image explanation:
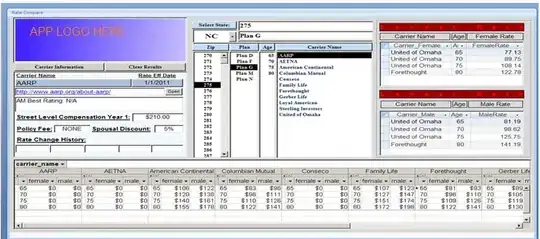
The left side shows the path you are taking, where you make a pointer go to the same blob, so both references edit the same blob. The right hand shows my answers, where you make a reference to a new blob in memory, and then edit that new blob. Please excuse my crude paint drawing, I'm no artist.