Nimbus doesn't operate like other look and feels (yea for us).
Normally you should be using a ColorUIResource
, but Nimbus prefers the use of Painter
s, for example...
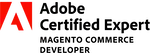
import java.awt.Color;
import java.awt.EventQueue;
import java.awt.GradientPaint;
import java.awt.Graphics2D;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.RenderingHints;
import javax.swing.JFrame;
import javax.swing.JProgressBar;
import javax.swing.Painter;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
import javax.swing.plaf.ColorUIResource;
public class TestNimbus {
public static void main(String[] args) {
new TestNimbus();
}
public TestNimbus() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel("com.sun.java.swing.plaf.nimbus.NimbusLookAndFeel");
ProgressPainter painter = new ProgressPainter(Color.WHITE, Color.GREEN);
UIManager.getLookAndFeelDefaults().put("ProgressBar[Enabled+Finished].foregroundPainter", painter);
//UIManager.getLookAndFeelDefaults().put("ProgressBar[Enabled+Finished].backgroundPainter", painter);
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
}
JFrame frame = new JFrame("Testing");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new GridBagLayout());
GridBagConstraints gbc = new GridBagConstraints();
gbc.gridwidth = GridBagConstraints.REMAINDER;
JProgressBar pb = new JProgressBar();
frame.add(pb, gbc);
pb = new JProgressBar();
pb.setValue(50);
frame.add(pb, gbc);
pb = new JProgressBar();
pb.setValue(100);
frame.add(pb, gbc);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
public class ProgressPainter implements Painter {
private Color light, dark;
private GradientPaint gradPaint;
public ProgressPainter(Color light, Color dark) {
this.light = light;
this.dark = dark;
}
@Override
public void paint(Graphics2D g, Object c, int w, int h) {
g.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
gradPaint = new GradientPaint((w / 2.0f), 0, light, (w / 2.0f), (h / 2.0f), dark, true);
g.setPaint(gradPaint);
g.fillRect(2, 2, (w - 5), (h - 5));
Color outline = new Color(0, 85, 0);
g.setColor(outline);
g.drawRect(2, 2, (w - 5), (h - 5));
Color trans = new Color(outline.getRed(), outline.getGreen(), outline.getBlue(), 100);
g.setColor(trans);
g.drawRect(1, 1, (w - 3), (h - 3));
}
}
}
I stole the painter from Custom Painter on JProgressBar, so credit there