If I understand your problem correctly, you have just run into the classic lock-ordering deadlock problem.
A program will be free of lock-ordering deadlocks if all threads
acquire the locks they need in a fixed global order. (from Java Concurrency in Practice)
If you detect in your data that different threads acquired the same locks in different order, then your program can potentially suffer a lock-ordering deadlock with unlucky timing. So that's the pattern to look for in your data; it's that simple.
UPDATE: Here is an algorithm how I would do it. I don't know whether it has a name.
For each lock event li
from the left to right:
find its corresponding unlock event (or use the end of the sequence if it is never released)
add all enclosed lock events as pairs (i,j)
where j
is an enclosed lock event
then go to the next lock event and repeat.
An example follows.
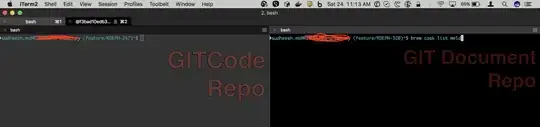
For example, for the first event lA
it means scanning through the sequence to find the first occurrence of uA
. This gives us the subsequence: lA lB uA
. For each lock event in this subsequence add a pair into a set. In this case, save (A,B)
in the set. (If we had another lock event in this subsequence, say
lA lB lD uA
, we would add the pair (A,D)
as well to the set.)
Now let's prepare the next subsequence. For the next lock event in the orgininal sequence, that is lB
, find the first uB
following it. This gives the subsequence is lB uA lC uB
and the only pair that needs to be saved in the set is (B,C)
.
For the third subsequence, for the lC
event there is no pair to be saved as there is no lock event in the lC uB
subsequence.
The set of thread 1
contains the pairs (A,B)
and (B,C)
. I would simple create another set containing the reversed pairs (B,A)
and (C,B)
; let's call it the forbidden set.
I would repeat this procedure for thread 2
, prepare the container with the pairs telling which locks were acquired in what order by thread 2
. Now, if the set intersection of this set and the forbidden set of thread 1
is not empty, then we have detected a potential lock-ordering deadlock.
Hope this helps.