As far I understand, pandas plot timeseries by using period values according to the frequency of your index. This makes sense because matplotlib only understands number as values for the axis and thus your call to broken_barh
fails because your are passing a non-number value.
To get the integer value of a period of a timestamp you need to use .to_period()
. See:
In [110]: pd.to_datetime('2014-04-02').to_period('D').ordinal
Out[110]: 16162
In [111]: pd.to_datetime('2014-04-02').to_period('W').ordinal
Out[111]: 2310
Then, depending on your timestamps interval (days, weeks, months, etc) you need to figure out what's the width you want to use for the broken bar.
In the example below, the frequency is 1 day and the width of the bar for one week is 7 units.
import numpy as np
import matplotlib.pylab as plt
import pandas as pd
idx = pd.date_range('2013-04-01', '2013-05-18', freq='D')
df = pd.DataFrame({'values': np.random.randn(len(idx))}, index=idx)
ax = df.plot()
start_period = idx[0].to_period('D').ordinal
bar1 = [(start_period, 7), (start_period + 10, 5), (start_period + 25, 4)]
bar2 = [(start_period, 1), (start_period + 22, 3), (start_period + 40, 2)]
ax.broken_barh(bar1, [2, .2], facecolor='red')
ax.broken_barh(bar2, [-2, .2], facecolor='green')
plt.show()
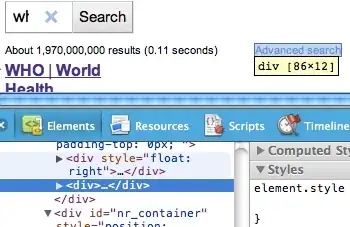