Here is a rather simple way assuming some first year calculus.
You can approximate functions by derivating them over and over and understanding their slope - and then building a polynomial around them such that the polynomial approximates their behavior well enough. If you keep doing this for as long as you can you get something called their taylor sequence. If a function is "well behaved" enough - such as the sine function, you can approximate it rather easily.
Here is the expansion of the sine function, taken from Wikipedia (CC wikipedia)
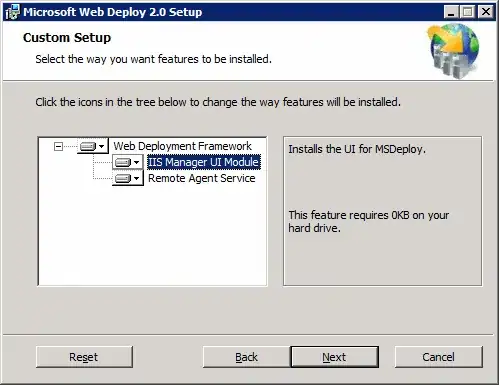
You can come up with this by derivating sin(x)
n times and approximating it. Read more on the subject here.
One helpful analysis it and come up with the inverse tangent function Math.atan
:
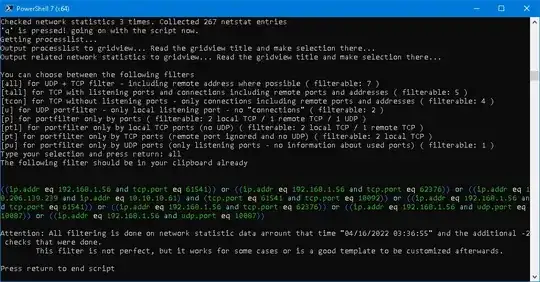
This is useful since putting x = 1
we know Math.atan(1) = Pi/4
.
So, let's write our getPi
:
function getPi(){
var sum = 0;
for(var n = 0; n < 100000000; n++){
var mult = (n%2 === 0) ? 1 : -1; // -1^n
sum += mult * (1 / (2*n+1));
}
return sum * 4; // to get pi
}
getPi(); // 3.141592643589326
The more iterations you perform, the better the accuracy you'll get. There are faster ways to calculate Pi, this is just an example that requires some - but not a huge amount of math. As mentioned - it works by approximating the atan function with a polynomial.
Note : we have bigger issues with larger numbers since JavaScript double precision numbers are bounded. We ignore that in this answer.