I've tried different ways to make it working, but unfortunetely it seems that this is not possible using OpenCV. The only thing you can do is probably display one window on main(primary) screen just using your current code and handle second window manually - set window position, resize image, and just use imshow
function to display it. Here is some example:
void showWindowAlmostFullscreen(cv::Mat img, std::string windowTitle, cv::Size screenSize, cv::Point screenZeroPoint)
{
screenSize -= cv::Size(100, 100); //leave some place for window title bar etc
double xScallingFactor = (float)screenSize.width / (float)img.size().width;
double yScallingFactor = (float)screenSize.height / (float)img.size().height;
double minFactor = std::min(xScallingFactor, yScallingFactor);
cv::Mat temp;
cv::resize(img, temp, cv::Size(), minFactor, minFactor);
cv::moveWindow(windowTitle, screenZeroPoint.x, screenZeroPoint.y);
cv::imshow(windowTitle, temp);
}
int _tmain(int argc, _TCHAR* argv[])
{
cv::Mat img1 = cv::imread("D:\\temp\\test.png");
cv::Mat img2;
cv::bitwise_not(img1, img2);
cv::namedWindow("img1", CV_WINDOW_AUTOSIZE);
cv::setWindowProperty("img1", CV_WND_PROP_FULLSCREEN, CV_WINDOW_FULLSCREEN);
cv::namedWindow("img2");
while(cv::waitKey(1) != 'q')
{
cv::imshow("img1", img1);
cv::setWindowProperty("img1", CV_WND_PROP_FULLSCREEN, CV_WINDOW_FULLSCREEN);
showWindowAlmostFullscreen(img2, "img2", cv::Size(1366, 768), cv::Point(260, 1080));
}
}
and the result:
Screen size and screen zero point (i don't know whether this is a correct name of this point - generally it's just a point in which there is screen (0,0) point) you can get using some other library or from windows control panel. Screen zero point will display when you will start moving screen:
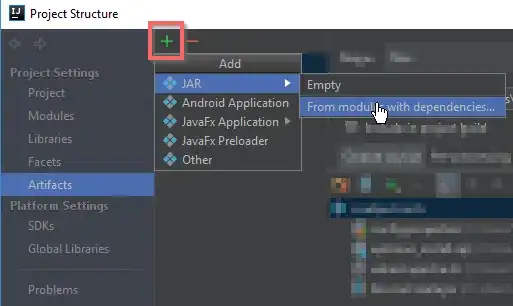