Solution by example
simpletest.rb
require "rubygems"
require "celerity"
word_to_search = ARGV[0]
word_to_check = ARGV[1]
#puts "DEBUG - word_to_search: #{word_to_search}"
#puts "DEBUG - word_to_check: #{word_to_check}"
browser = Celerity::Browser.new
browser.goto('http://www.google.com')
browser.text_field(:name, 'q').value = word_to_search
browser.button(:name, 'btnG').click
puts browser.text.include? word_to_check
Suppose we have to interface Excel 2011 on a Mac with this simple script that takes a couple of input parameters and returns a result
test.sh
#!/bin/bash --login
rvm use jruby > NULL
ruby simpletest.rb $1 $2
We write a shell you can invoke via VBA code;
Please be aware to set proper permission chmod u+x test.sh
The shebang: #!/bin/bash --login
this is required in order to run rvm
to select jruby ( that is the only ruby supported by celerity
VBA Module ( User Defined Function )
Public Function SimpleTest(sWordToSearch, sWordToCheck)
Dim sCommand As String
sCommand = "do shell script ""cd /Users/ronda/projects/so-test1; ./test.sh "
sCommand = sCommand & sWordToSearch & " "
sCommand = sCommand & sWordToCheck & """"
SimpleTest = MacScript(sCommand)
End Function
Note in this code: /Users/ronda/projects/so-test1
is the path to our scripts
Usage & Testing
Testing the User Defined Function in a formula
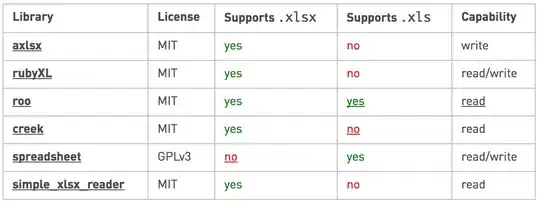
Btw the diagnosis of problems may be streamlined by running the script to a lower level:
Testing in the AppleScript edtor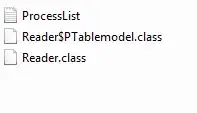
Testing the shell script in terminal
$ cd ~/projects/so-test1
$ ./test.sh Ruby Language
true
$ ./test.sh Fish Language
false
Testing the jruby script standalone
$ rvm use jruby
Using /Users/ronda/.rvm/gems/jruby-1.7.11
$ ruby simpletest.rb Ruby Language
true
$ ruby simpletest.rb Ruby Fish
false
further reading
Just a couple of useful link:
- read this answer to How do I issue an HTTP GET from Excel VBA for Mac 2011 as an alternative (possibly the most powerful) approach using popen and pipes
- Accessing Your Custom
Functions