How to hook an HTTP Module into an MVC application
If I understand you correctly, you want to create an external library and "hook" it up to an MVC application's events.
1. Create a simple class library.
The first thing is to create a simple class library. We'll call it TestLib
.

2. Create a new class called TestLibHttpModule
.
The class implements IHttpModule
. This grants it the Init()
method. This method will be called when the module is initialised and it passes us the HttpApplication
object that is initialising the module.
In our Init
method, we'll attach a new EventHandler
to the EndRequest
event.
For now, our event handler method will simply throw an exception with a cheeky message.
namespace TestLib
{
public class TestLibHttpModule : IHttpModule
{
public void Dispose()
{
}
public void Init(HttpApplication context)
{
context.EndRequest += new EventHandler(context_EndRequest);
}
private void context_EndRequest(object sender, EventArgs e)
{
// get the HttpApplication context
HttpApplication context = (HttpApplication)sender;
throw new NotImplementedException("At least it works.");
}
}
}
3. Point the library's build path to our MVC project.
Assuming you have an MVC project already set up nearby, perhaps called TestApp, point the build path of your library to be the bin
folder of your MVC project. Now, every time we build the module, it'll be thrown into the MVC project.
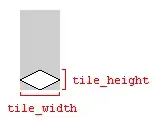
4. Update MVC project to use the HttpModule.
The Web.config of an MVC application has a spot for specifying Http Modules. Under the system.Web
element, add a new httpModules
section (if it doesn't already exist).
<system.web>
[ ... ]
<httpModules>
<add name="TestLibModule" type="TestLib.TestLibHttpModule, TestLib" />
</httpModules>
</system.web>
5. Run the MVC application.
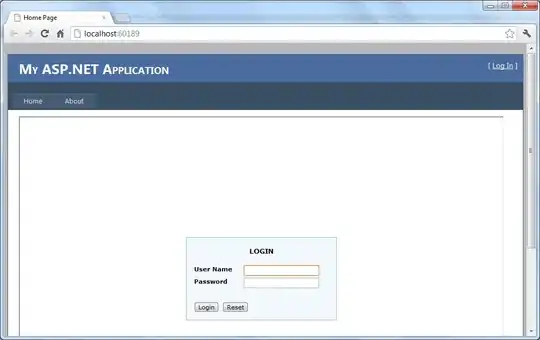