Depended on the platform, the name of Times New Roman font can be different, for example in my current machine, Mac OS
:
import matplotlib.font_manager as fmg
for i, item in enumerate(fmg.findSystemFonts()):
try:
name=fmg.FontProperties(fname=item).get_name()
if 'TIMES' in name.upper():
print name, item, i
except:
pass
#Times New Roman /Library/Fonts/Times New Roman Italic.ttf 223
#Times New Roman /Library/Fonts/Times New Roman Bold Italic.ttf 232
#Times New Roman /Library/Fonts/Times New Roman Bold.ttf 336
#Times New Roman /Library/Fonts/Times New Roman.ttf 367
I think this solution may be safer: directly supply the path to the font file. matplotlib.font_manager.findSystemFonts()
will list the paths to every file:
x = np.array([0, 75, 150])
y = np.array([0, 1, 3])
coeff = np.polyfit(x, y, 2)
xx = np.linspace(0, 150, 150)
yy = coeff[0] * xx**2 + coeff[1] * xx + coeff[2]
all_font = fmg.findSystemFonts()
fontprop = fmg.FontProperties(fname=all_font[223]) #you may have a different index
plt.title(unicode("Correction factor", "utf-8"), fontproperties=fontprop)
fontprop = fmg.FontProperties(fname=all_font[232])
plt.xlabel(unicode("Temperature in °C", "utf-8"), fontproperties=fontprop)
fontprop = fmg.FontProperties(fname=all_font[236])
plt.ylabel(unicode("fKorr", "utf-8"), fontproperties=fontprop)
plt.plot(xx,yy)
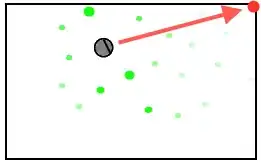