No need for epensive MATLAB, you can do that really easily in ImageMagick which is free and available here
So, basically you use this command:
convert yourpicture.jpg -colorspace rgb -colors 256 -depth 8 txt:
and it gives you this - a listing of all the pixels and their values:
# ImageMagick pixel enumeration: 32,32,255,rgb
0,0: (255,255,0) #FFFF00 rgb(255,255,0)
1,0: (255,255,0) #FFFF00 rgb(255,255,0)
2,0: (255,255,0) #FFFF00 rgb(255,255,0)
Then you can get rid of all the superfluous stuff and just look at the RGB values like this:
convert yourimage.jpg -colorspace rgb -colors 256 -depth 8 txt: | \
awk -F'[()]' '/^[0-9]/{print $4}' | \
awk -F, '{R=$1;G=$2;B=$3; print R,G,B}'
Sample Output
0 255 255
0 255 255
8 156 8
8 156 8
0 55 0
0 55 0
0 55 0
8 156 8
The above code will take your image, whether JPEG or PNG or TIFF and list all the RGB values of all the pixels.
If we now assume gold is RGB 255,215,0 we can do this:
# Find gold pixels +/- fuzz factor of 25
#
convert yourpicture.jpg -colorspace rgb -colors 256 -depth 8 txt: | \
awk -F'[()]' '/^[0-9]/{print $4}' | \
awk -F, 'BEGIN {gold=0}
{R=$1;G=$2;B=$3; if((R>230) && (G>190) && (G<240) && (B<25))gold++}
END {print "Gold pixels found: ",gold}'
If you want to work with hue/saturation and value, you can equally do that in ImageMagick like this:
# Find gold pixels +/- fuzz Hue=14
#
convert yourpicture.jpg -colorspace hsv -depth 8 txt:| \
awk -F'[()]' '/^[0-9]/{print $4}' | \
awk -F, 'BEGIN {gold=0}
{H=$1; if((H>11)&&(H<16))gold++}
END {print "Gold pixels found: ",gold}'
I should point out that the range of Hue is 0-360, so if we search for 14 (+/- fuzz) we are looking for a Hue of 14% of 360, i.e. Hue=50 which corresponds to gold.
If you want to visualise which pixels are selected and counted, you can do this:
convert pcb.jpg -channel R -fx "hue>0.09&&hue<0.11?1:0" -separate -background black -channel R -combine pcb_gold.jpg
which, given this input image, will produce this result:
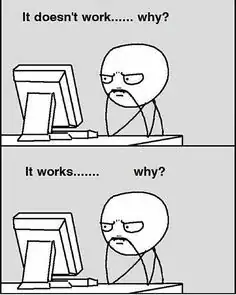
Even easier than the foregoing, is this little script that does all you want!
#!/bin/bash
# Define our gold colour
#
gold="rgb(255,215,0)"
# Convert all pixels in the image that are gold +/-35% into red. Write output to "out.jpg"
# ... and also in text format so we can count the red pixels with "grep -c"
convert pcb.jpg -fuzz 35% -fill red -opaque "$gold" -depth 8 -colorspace rgb -write txt: out.jpg | grep -c FF0000
which produces this image, and the gold pixel count of 1076.
