But console.log(formData)
shows formData
is empty.
What do you mean by "empty"? When I test this in Chrome it shows ‣ FormData {append: function}
... which is to say it's a FormData object, which is what we expected. I made a fiddle and expanded to code to this:
var form = document.querySelector('form'),
formData = new FormData(form),
req = new XMLHttpRequest();
req.open("POST", "/echo/html/")
req.send(formData);
...and this is what I saw in the Chrome Developer Tools Network panel:
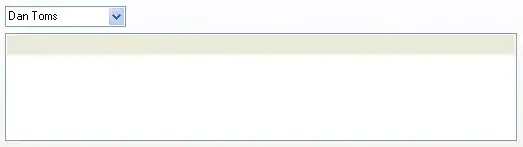
So the code is working as expected.
I think the disconnect here is that you're expecting FormData to work like a vanilla JavaScript object or array and let you directly look at and manipulate its contents. Unfortunately it doesn't work like that—FormData only has one method in its public API, which is append
. Pretty much all you can do with it is create it, append values to it, and pass it to an XMLHttpRequest.
If you want to get the form values in a way you can inspect and manipulate, you'll have to do it the old-fashioned way: by iterating through the input elements and getting each value one-by-one—or by using a function written by someone else, e.g. jQuery's. Here's an SO thread with a few approaches to that: How can I get form data with JavaScript/jQuery?