I solved this myself using a container RelativeLayout as an anchor, with the parent button and all child buttons given android:layout_centerInParent
. Then, to solve the issue of the child buttons disappearing when leaving the container, I gave the container android:clipChildren(false)
and also set clipChildren
to false
on all of its ancestors as well.
Note that the container has to be bigger than all of its child elements, or all the child elements will be clipped to the same dimensions, even when they move outside of the container! To solve this, I gave the container a width and height of wrap_content
.
Thus, all my child buttons were centered in the parent button no matter where I positioned the parent, and the child buttons were free to move around as well.
EDIT
A major flaw in this is that buttons can't recieve touch events if they are outside of their parent. To fix this, you can either use event coordinates or make the parent container big enough to always encompass the child elements (maybe twice the screen width/height?)
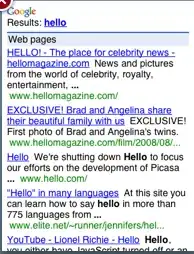
Here is the code:
res/layout/listfragment.xml
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:clipChildren="false" >
<ListView
android:id="@android:id/list"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<RelativeLayout
android:onClick="onButterflyMenuClicked"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|right"
android:layout_marginBottom="20sp"
android:layout_marginRight="15sp"
android:clipChildren="false" >
<Button
android:id="@+id/btn_north_1"
style="@style/PeekabooButton"
android:text="1st"
android:translationY="-65sp" />
<Button
android:id="@+id/btn_north_2"
style="@style/PeekabooButton"
android:text="2nd"
android:translationY="-115sp" />
<Button
android:id="@+id/kingbutton"
android:layout_width="65sp"
android:layout_height="65sp"
android:layout_centerInParent="true"
android:gravity="center_vertical|center_horizontal"
android:textSize="16sp"
android:text="KING" />
</RelativeLayout>
</FrameLayout>
res/values/styles.xml
<style name="PeekabooButton">
<item name="android:layout_width">45sp</item>
<item name="android:layout_height">45sp</item>
<item name="android:layout_centerInParent">true</item>
<item name="android:textSize">10sp</item>
</style>