I think much easier would be to write your own function
To find (perpendicular) distance between point and line and then creating thickness of poly-line by polygon means.
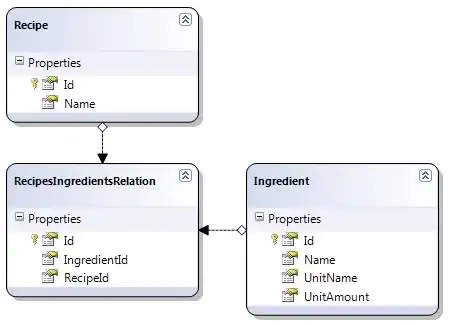
Where:
P0,P1
are line endpoints
P
is point
d
is distance between them
Line is defined as: p(t)=P0+(P1-P0)*t
where t=<0.0,1.0>
So the function should do this:
create perpendicular line
q(t)=P+DQ*u
where u=(-inf,+inf)
DQ
is perpendicular vector to (P1-P0)
In 2D you can obtain it easily like this (x,y) -> (y,-x)
. In higher dimensions use cross product with some non coplanar vectors.
compute line vs. line intersection
there are tons of stuff about this so google or solve the equation yourself here you can extract mine implementation.
now after successful intersection
just compute d
as distance between P
and intersection point. Do not forget that parameter t
must be in range. If not (or if no intersection) then return min(|P-P0|,|P-P1|)
[hints]
t
and u
parameters can be obtained directly from intersection test so if the perpendicular vector to (P1-P0)
is normalized to size = 1
then the abs(u)
parameter of intersection point is the distance
[notes]
I am not familiar with mongodb so if you have no means to use own tests inside then this answer is of coarse obsolete.