Ok, here’s the code to make this:
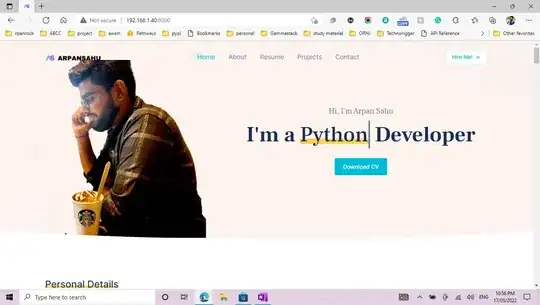
The above picture has 2 rows of buttons: one on top and one on the bottom. In the middle is the WebView.
Here is my GitHub account where u can download the source code:
https://github.com/GeneChuang1/Android/tree/Android
Otherwise, here is the App breakdown:
The Java Code (AndroidMobileAppSampleActivity.java):
package com.gene;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.webkit.WebSettings;
import android.webkit.WebView;
import android.webkit.WebViewClient;
import com.gene.R;
public class AndroidMobileAppSampleActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.app_page);
WebView mainWebView = (WebView) findViewById(R.id.webcontent);
WebSettings webSettings = mainWebView.getSettings();
webSettings.setJavaScriptEnabled(true);
mainWebView.setWebViewClient(new MyCustomWebViewClient());
mainWebView.setScrollBarStyle(View.SCROLLBARS_INSIDE_OVERLAY);
mainWebView.loadUrl("http://www.google.com");
}
private class MyCustomWebViewClient extends WebViewClient {
@Override
public boolean shouldOverrideUrlLoading(WebView view, String url) {
view.loadUrl(url);
return true;
}
}
}
I have 2 XML layouts. One for the main webpage, the other is a pre-made menu that I in the main webpage.
The XML Layout “app_page.xml”:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<LinearLayout
android:id="@+id/page_weekly_items_options_menu"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:background="#d4dbe1"
android:gravity="center"
android:orientation="horizontal">
<ImageView
android:id="@+id/share"
android:layout_width="60dp"
android:layout_height="50dp"
android:background="@drawable/icon"
android:clickable="true"
android:onClick="userClickedShare"></ImageView>
<ImageView
android:id="@+id/left_arrow"
android:layout_width="60dp"
android:layout_height="50dp"
android:background="@drawable/icon"
android:clickable="true"
android:onClick="userClickedLeftArrow"></ImageView>
<ImageView
android:id="@+id/right_arrow"
android:layout_width="60dp"
android:layout_height="50dp"
android:background="@drawable/icon"
android:clickable="true"
android:onClick="userClickedRightArrow"></ImageView>
<ImageView
android:id="@+id/notifications_pageweeklyitem"
android:layout_width="60dp"
android:layout_height="50dp"
android:background="@drawable/icon"
android:clickable="true"
android:onClick="userClickedNotificationsPageWeeklyItem"></ImageView>
<ImageView
android:id="@+id/favorites_pageweeklyitem"
android:layout_width="60dp"
android:layout_height="50dp"
android:background="@drawable/icon"
android:clickable="true"
android:onClick="userClickedFavoritesPageWeeklyItem"></ImageView>
</LinearLayout>
<RelativeLayout
android:id="@+id/webcontent_container"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_below="@id/page_weekly_items_options_menu">
<WebView
android:id="@+id/webcontent"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_above="@+id/menu"
></WebView>
<include
android:id="@+id/menu"
layout="@layout/bottom_menu"
android:layout_height="wrap_content"
android:layout_width="match_parent"
android:layout_alignParentBottom="true"
android:gravity="bottom"
android:layout_weight="1"
/>
</RelativeLayout>
</RelativeLayout>
The other XML layout is “bottom_menu.xml”:
<HorizontalScrollView xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/bottom_scroll_menu"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="bottom" >
<!-- This layout is used by activity_main.xml.
It is part of the main home page -->
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="#17528c"
android:orientation="horizontal" >
<ImageView
android:id="@+id/Weekly"
android:layout_width="60dp"
android:layout_height="50dp"
android:background="@drawable/icon"
android:clickable="true"
android:onClick="userClickedWeekly" >
</ImageView>
<ImageView
android:id="@+id/Search"
android:layout_width="60dp"
android:layout_height="50dp"
android:background="@drawable/icon"
android:clickable="true"
android:onClick="userClickedSearch" >
</ImageView>
<ImageView
android:id="@+id/Favorites"
android:layout_width="60dp"
android:layout_height="50dp"
android:background="@drawable/icon"
android:clickable="true"
android:onClick="userClickedFavorites" >
</ImageView>
<ImageView
android:id="@+id/Notifications"
android:layout_width="60dp"
android:layout_height="50dp"
android:background="@drawable/icon"
android:clickable="true"
android:onClick="userClickedNotifications" >
</ImageView>
<ImageView
android:id="@+id/Profile"
android:layout_width="60dp"
android:layout_height="50dp"
android:background="@drawable/icon"
android:clickable="true"
android:onClick="userClickedProfile" >
</ImageView>
<ImageView
android:id="@+id/About"
android:layout_width="60dp"
android:layout_height="50dp"
android:background="@drawable/icon"
android:clickable="true"
android:onClick="userClickedAbout" >
</ImageView>
</LinearLayout>
</HorizontalScrollView>
The Android Manifest (just incase someone forgets the internet permission):
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="tscolari.mobile_sample"
android:versionCode="1"
android:versionName="1.0">
<uses-sdk android:minSdkVersion="10" />
<uses-permission android:name="android.permission.INTERNET"/>
<application android:icon="@drawable/icon" android:label="@string/app_name">
<activity android:name=".AndroidMobileAppSampleActivity"
android:label="@string/app_name">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Again, here is my GitHub account where u can download the source code:
https://github.com/GeneChuang1/Android/tree/Android
-Gene Chuang