Dietrich
gave you a nice answer, but if you want to keep all the functionality (non-base 10, non-integer exponents) of LogFormatter
, then you might create your own formatter:
import matplotlib.ticker
import matplotlib
import re
# create a definition for the short hyphen
matplotlib.rcParams["text.latex.preamble"].append(r'\mathchardef\mhyphen="2D')
class MyLogFormatter(matplotlib.ticker.LogFormatterMathtext):
def __call__(self, x, pos=None):
# call the original LogFormatter
rv = matplotlib.ticker.LogFormatterMathtext.__call__(self, x, pos)
# check if we really use TeX
if matplotlib.rcParams["text.usetex"]:
# if we have the string ^{- there is a negative exponent
# where the minus sign is replaced by the short hyphen
rv = re.sub(r'\^\{-', r'^{\mhyphen', rv)
return rv
The only thing this really does is to grab the output of the usual formatter, find the possible negative exponents and change the LaTeX code of the math minus into something else. Of course, if you invent some creative LaTex with \scalebox
or something equivalent, you may do so.
This:
import matplotlib.pyplot as plt
import numpy as np
matplotlib.rcParams["text.usetex"] = True
fig = plt.figure()
ax = fig.add_subplot(111)
ax.semilogy(np.linspace(0,5,200), np.exp(np.linspace(-2,3,200)*np.log(10)))
ax.yaxis.set_major_formatter(MyLogFormatter())
fig.savefig("/tmp/shorthyphen.png")
creates:
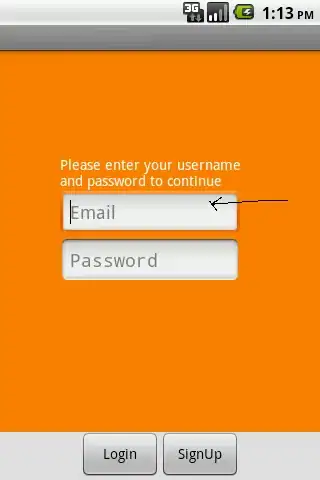
The good thing about this solution is that it changes the output as minimally as possible.