Your program has likely encountered what's called catastrophic backtracking.
If you have a bit of time, let's look at how your regex works...
Quick refresher: How regex works: The state machine always reads from left to right, backtracking where necessary.
On the left hand side, we have our pattern:
/^([a-zA-Z]+ *)+$/
And here's the String to match:
dssdfsdfdsf wdasdads dadlkn mdsds .
From the regex101 debugger, your regex took 78540 steps to fail. This is because you used quantifiers that are greedy and not possessive (backtracking).
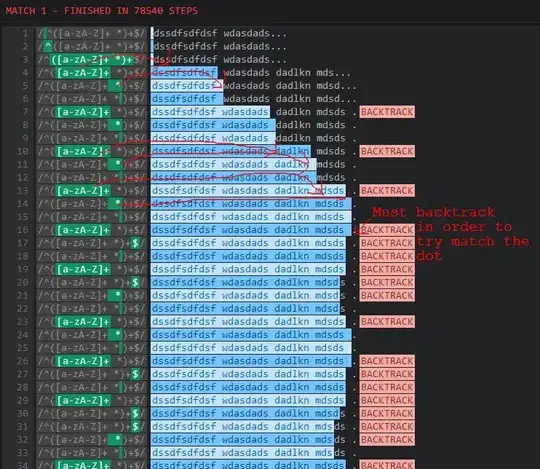
... Long story short, because the input string fails to match, every quantifier within your regex causes indefinite backtracking - Every character is released from +
and then *
and then both and then a group is released from ()+
to backtrack more.
Here's a few solutions you should follow:
Avoid abundant quantifiers!
If you revise your expression, you'll see that the pattern is logically same as:
/^[a-zA-Z]+( +[a-zA-Z]+)*$/
This uses a step of logical induction to reduce the regex upstairs to match far quicker, now at 97 steps!

Use possessive quantifiers while you can!
As I mentioned, /^([a-zA-Z]+ *)+$/
is evil because it backtracks in a terrible manner. We're in Java, what can we do?
This solution works only because [a-zA-Z]
and
matches distinct items. We can use a possessive group!
/^([a-zA-Z]++ *+)++$/
^ ^ ^
These simple "+
" denotes "We're not backtracking if we fail the match from here". This is an extremely effective solution, and cuts off any need for backtracking. Whenever you have two distinct groups with a quantifier in between, use them. And if you need some proof on the effectiveness, here's our scorecard:
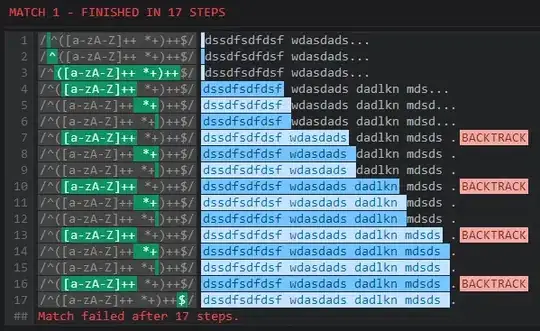
Read also:
Online Demos: