There are different methods you can use depending on the context and your needs, but I tried out the answers from i3arnon and alltej, and in debugging I found that they both have the same problem... I ran both code samples in a console app I made. I set a break point in the code and found that on the line of execution with Task.WaitAll
or Task.WhenAll
. When I examined the tasks I found that they had already run - the task property Status
was = ran to completion
. In fact, I was able to completely remove the Task.
line of code and still assign the variables values, so it was useless.
I found it was a very difficult task to truly make this kind of operation run as desired and also get the return values needed. Here is what I came up with:
Task[] taskArray = {
Task.Factory.StartNew(() => ExecuteConcurrentTasks(1).Result),
Task.Factory.StartNew(() => ExecuteConcurrentTasks(2).Result)
};
Task.WaitAll(taskArray);
var s = ((Task<string>)taskArray[0]).Result;
This is a simple example where a task array is created, then Task.WaitAll
is used to execute the 2 tasks simultaneously, then in the last line I'm showing how to access the returned value from the first task.
Here is the code with some more context and more data:
private static async void MyAsyncMethod()
{
Task[] taskArray = {
Task.Factory.StartNew(() => ExecuteConcurrentTasks(1).Result),
Task.Factory.StartNew(() => ExecuteConcurrentTasks(2).Result),
Task.Factory.StartNew(() => ExecuteConcurrentTasks(3).Result),
Task.Factory.StartNew(() => ExecuteConcurrentTasks(4).Result)
};
Task.WaitAll(taskArray);
string s1 = ((Task<string>)taskArray[0]).Result;
string s2 = ((Task<string>)taskArray[1]).Result;
string s3 = ((Task<string>)taskArray[2]).Result;
string s4 = ((Task<string>)taskArray[3]).Result;
}
private static async Task<string> ExecuteConcurrentTasks(int passedInt)
{
string s = "Result: " + passedInt.ToString();
if (passedInt == 4)
{
Console.WriteLine(s);
}
else if (passedInt == 3)
{
Console.WriteLine(s);
}
else if (passedInt == 2)
{
Console.WriteLine(s);
}
else if (passedInt == 1)
{
Console.WriteLine(s);
}
return s;
}
Here is the output:
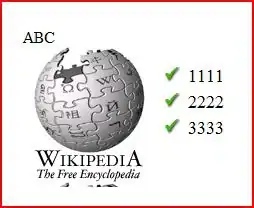
UPDATE:
I got a bit more insight on these methods from working with some other developers. My understanding now is that .WaitAll
will block execution in other parts of the program, which is an issue if you're not running just a very simple program. Also .WhenAll
doesn't mean that the tasks won't execute until that line and all begin asynchronous execution at that line, it just means that the code in that block won't continue beyond that point unless all the specified tasks have finished running.