Should I be using a different layout?
No, stick with MigLayout
. You have chosen the right layout manager.
I advice you to invest some time into learning this manager; create couple
of smaller practical examples, learn the numerous constraints that this
manager provides.
It also puts my above buttons out of place and I'm not too sure why.
MigLayout
is a grid-based layout manager. (Its most important mode is.)
The gap between the New and Open buttons is created because the
highlighted components and the New button form a column. The column width
is determined by the width of the largest cell. To bypass this, we can
use the split
constraint. (Often used in combination with span
constraint.)
In my example I use this technique to center two buttons above the
currently visible label.
If we are not sure about something in the layout, we can use the
debug
layout constraint which paints the lines of the grid and bounds
of the components.
frame.getContentPane().setLayout(new MigLayout("", "[53px,grow][57px][grow]", "[23px][][][]"));
Do not set bounds in pixels. You are not utilizing of one of the
most important advantages of this manager -- independence of resolution and
DPI. Bounds in pixels are not portable. A 3px gap between buttons looks OK on a smaller screen but is not acceptable on a larger one.
MigLayout
provides several options to choose from including related and unrelated gaps, logical pixels, points, or centimeters.
itemTitle.setMinimumSize(new Dimension(50, 50));
Setting a minimum size of a component is usually not necessary. But if we need to
do it, we have the wmin
and wmax
constraints. This should be done by the
layout manager, not by code outside of it. The set(Minimum|Maximum|Preferred)size
methods should be avoided. (With poorer managers, one cannot do without them, however.)
And again, setting dimensions in pixels is not optimal.
Now we get to the solution. MigLayout
has hidemode
constraint to deal
with your requirement. There are four hide modes. I assume that we need
the hidemode 3
, in which all invisible components do not participate in the
layout.
package com.zetcode;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.util.ArrayList;
import javax.swing.AbstractAction;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import net.miginfocom.swing.MigLayout;
public class HidingComponentsEx extends JFrame {
private ArrayList<JLabel> lbls;
private int itemVisible = 0;
public HidingComponentsEx() {
initUI();
}
private void initUI() {
createLabels();
JButton prevBtn = new JButton("Previous");
prevBtn.addActionListener(new PrevAction());
JButton nextBtn = new JButton("Next");
nextBtn.addActionListener(new NextAction());
JPanel pnl = new JPanel(new MigLayout("ins dialog"));
pnl.add(prevBtn, "split 2, center");
pnl.add(nextBtn, "wrap");
for (JLabel lbl : lbls) {
pnl.add(lbl, "cell 0 1, w 250lp, h 100lp, hidemode 3");
}
add(pnl);
pack();
setTitle("MigLayout example");
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private void createLabels() {
lbls = new ArrayList<>();
lbls.add(createLabel("North Sea"));
lbls.add(createLabel("Ionian Sea"));
lbls.add(createLabel("Norwegian Sea"));
lbls.add(createLabel("Bering Sea"));
lbls.add(createLabel("Dead Sea"));
lbls.get(itemVisible).setVisible(true);
}
private class NextAction extends AbstractAction {
@Override
public void actionPerformed(ActionEvent e) {
lbls.get(itemVisible).setVisible(false);
itemVisible++;
if (itemVisible > 4) {
itemVisible = 0;
}
lbls.get(itemVisible).setVisible(true);
}
}
private class PrevAction extends AbstractAction {
@Override
public void actionPerformed(ActionEvent e) {
lbls.get(itemVisible).setVisible(false);
itemVisible--;
if (itemVisible < 0) {
itemVisible = 4;
}
lbls.get(itemVisible).setVisible(true);
}
}
private JLabel createLabel(String text) {
JLabel lbl = new JLabel(text, JLabel.CENTER);
lbl.setVisible(false);
lbl.setBorder(BorderFactory.createEtchedBorder());
return lbl;
}
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
HidingComponentsEx ex = new HidingComponentsEx();
ex.setVisible(true);
}
});
}
}
Our example has two buttons and five labels. The buttons dynamically change their
visibility.
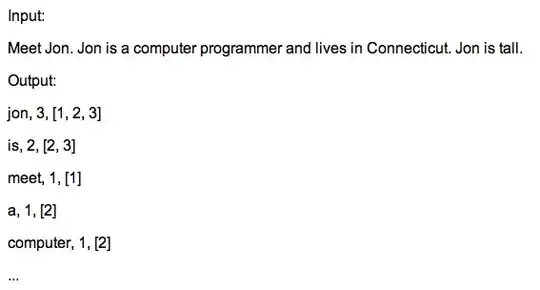