Here's your savior! (5 years later)
Use a SQL Function!
What the following code do?
The function fn_split_string_to_column
takes the list using a common separator and returns a table
CREATE FUNCTION [dbo].[fn_split_string_to_column] (
@string NVARCHAR(MAX),
@delimiter CHAR(1)
)
RETURNS @out_put TABLE (
[column_id] INT IDENTITY(1, 1) NOT NULL,
[value] NVARCHAR(MAX)
)
AS
BEGIN
DECLARE @value NVARCHAR(MAX),
@pos INT = 0,
@len INT = 0
SET @string = CASE
WHEN RIGHT(@string, 1) != @delimiter
THEN @string + @delimiter
ELSE @string
END
WHILE CHARINDEX(@delimiter, @string, @pos + 1) > 0
BEGIN
SET @len = CHARINDEX(@delimiter, @string, @pos + 1) - @pos
SET @value = SUBSTRING(@string, @pos, @len)
INSERT INTO @out_put ([value])
SELECT LTRIM(RTRIM(@value)) AS [column]
SET @pos = CHARINDEX(@delimiter, @string, @pos + @len) + 1
END
RETURN
END
SELECT * FROM empTable WHERE empCode IN (@employeeCode) -- this wont work!
SELECT * FROM empTable WHERE empCode IN (
select value from fn_split_string_to_column(@employeeCode,',') -- this will work!
)
Examples!
- Using comma as separator
declare @txt varchar(100) = 'a,b,c, d, e'
select * from fn_split_string_to_column(@txt,',')
Query results:
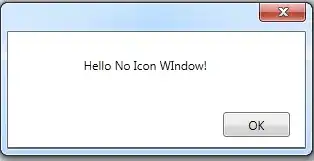
- Using space as separator
declare @txt varchar(100) = 'Did you hear about the guy who lost his left arm and left leg in an accident? He’s all right now.'
select * from fn_split_string_to_column(@txt,' ')
Query results:
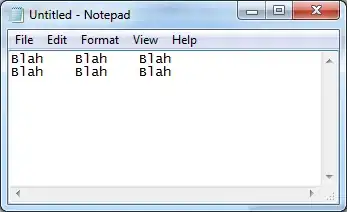
Source
The function above is not mine. I borrowed from the answer of the following question:How to split a comma-separated value to columns
Go there and give him an upvote!
(Actually you should go there and read the comments about performance issues you should know about)