You can achieve this by adding a handle to the DocumentCompleted event and use LINQ to query the HtmlDocument.
Example:
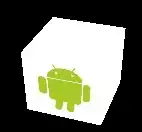
Option Strict On
Public Class Form1
Public Sub New()
Me.InitializeComponent()
Me.Button1 = New Button() With {.TabIndex = 0, .Dock = DockStyle.Top, .Text = "Load web page", .Height = 30}
Me.WebBrowser1 = New WebBrowser() With {.TabIndex = 1, .Dock = DockStyle.Fill}
Me.ComboBox1 = New ComboBox() With {.TabIndex = 2, .Dock = DockStyle.Bottom, .DropDownStyle = ComboBoxStyle.DropDownList}
Me.Controls.AddRange({Me.ComboBox1, Me.WebBrowser1, Me.Button1})
End Sub
Private Sub HandleButtonClick(sender As Object, e As EventArgs) Handles Button1.Click
Me.WebBrowser1.DocumentText = <!--
<!DOCTYPE html>
<html>
<body>
<select name="selectName">
<option value="1">Value 1 text</option>
<option value="2">Value 2 text</option>
<option value="3">Value 3 text</option>
<option value="4">Value 4 text</option>
</select>
</body>
</html>
-->.Value
End Sub
Private Sub HandleWebBrowserDocumentCompleted(sender As Object, e As WebBrowserDocumentCompletedEventArgs) Handles WebBrowser1.DocumentCompleted
If (Not Me.WebBrowser1.Document Is Nothing) Then
Dim selectTag As HtmlElement = (
From
element As HtmlElement
In
Me.WebBrowser1.Document.GetElementsByTagName("select").Cast(Of HtmlElement)()
Where
element.Name = "selectName"
Select
element
).FirstOrDefault()
If (Not selectTag Is Nothing) Then
Dim options As List(Of KeyValuePair(Of Integer, String)) = (
From
element As HtmlElement
In
selectTag.GetElementsByTagName("option").Cast(Of HtmlElement)()
Select
New KeyValuePair(Of Integer, String)(Integer.Parse(element.GetAttribute("value")), element.InnerText)
).ToList()
Me.ComboBox1.DataSource = options
Me.ComboBox1.ValueMember = "Key"
Me.ComboBox1.DisplayMember = "Value"
End If
End If
End Sub
Private WithEvents Button1 As Button
Private WithEvents WebBrowser1 As WebBrowser
Private WithEvents ComboBox1 As ComboBox
End Class